1. Overview
The Encap REST API is used to integrate client systems with the Encap server in order to perform mobile authentication of customers via designated mobile applications that integrate the Encap mobile authentication API.
Operations provided via the API are currently divided into the following categories:
-
Enrollment: Operations facilitating the enrollment of a supported application instance on a customer’s mobile device with the Encap server. The enrollment process creates a very strong cryptographic binding between the device/application instance and the server which can then be used to perform authentication operations.
-
Authentication: Operations for executing secure mobile authentication requests via previously-enrolled device/application instances.
-
Device Management: Operations for the management of enrolled device/application instances, including locking/unlocking of instances and deactivation/un-enrollment.
-
Admin APIs: Administrative checks and operations.
2. Common concepts
2.1. REST API authentication
Authentication for REST API calls (not to confuse with the authentication service itself) are handled by using Basic Authentication. All requests are required to have an Authorization header containing a Basic Authentication string. The Basic Authentication should have the API key id as username and the API key secret as password. API key provisioning is handled by the Encap server administrator.
GET /api/smart-device/v1/authentications/cd7cc4ad-4def-4555-b859-6791826d7665 HTTP/1.1
Authorization: Basic MTQ4NzRjZjItYzBiMC00YWYyLThjOTYtNWNmNTYxNDEzOTYxOjFzVk4vY0tSTlJaSmcxQWJDQkNmRmc9PQ==
Host: localhost:8080
2.1.1. Authentication error response
During authentication there are two errors that can occur
400 Bad Request is returned if the request is missing the required authentication headers.
401 Unauthorized is returned when authentication fails due to wrong/invalid API key id or API key secret.
2.2. HTTP verbs
The API is implemented to adhere as closely as possible to HTTP standard and REST conventions in its use of HTTP verbs.
Verb | Usage |
---|---|
|
Used to retrieve a resource. |
|
Used to create a new resource. |
|
Full update of an existing resource. |
|
Partial update of an existing resource. |
|
Used to delete an existing resource. |
2.3. HTTP status codes
The API is implemented to adhere as closely as possible to HTTP standard and REST conventions in its use of HTTP status codes.
Status code | Usage |
---|---|
|
The request completed successfully |
|
A new resource has been created successfully. The resource’s URI is available from the response’s
|
|
An update to an existing resource has been applied successfully |
|
The request was malformed. The response body will include an error providing further information. |
|
Authentication/Authorization failed for the request |
|
Client not authorized to access the operation on the specified resource |
|
Resource with the given id does not exist |
|
Tried to create a resource that already exists |
|
Conditional request, but conditions not fulfilled |
|
Content well formed but content validation failed |
|
Conditional request required but no condition given |
|
Unexpected failure on server side |
2.4. Errors
The API uses HTTP status codes to indicate the success or failure of an API request. In general, codes in the 2xx range indicate success, codes in the 4xx range indicate an error that failed given the request data provided (e.g., a required parameter was omitted, a device state not appropriate, etc.), and codes in the 5xx range indicate an error with the API servers.
Not all errors map cleanly onto HTTP response codes. Some 4xx codes also contain an error entity in the response explaining the error in more detail. In very rare cases HTTP 500 will do the same.
2.4.1. The error object
The error entity has the following response structure:
Field | Type | Description |
---|---|---|
|
|
Holds a duplicate copy of the HTTP error status code for convenience, so that clients can “see” it without having to analyze the response’s header |
|
|
Unique code for this specific error type. More fine grained than the HTTP code, e.g. "AUTH_LEVEL_NOT_AVAILABLE_FOR_DEVICE" |
|
|
Short description of the error, what might have cause it and possibly a “fixing” proposal, e.g. "Device is not activated for 2FA authentication, activate the device for 2FA before trying to use it for 2FA authentication" |
|
|
The correlation ID sent in the request or generated on the server. Will contain the same value as the response header X-Correlation-UUID. Can be used to find the correlating error messages from the server log |
|
|
Detailed message, containing additional data that might be relevant to the developer. Only provided when development mode is switched on and can potentially contain stack trace information or similar |
|
|
Array of field specific errors if there are any, typically used for validation faults (HTTP 422) |
Field specific error
Field | Type | Description |
---|---|---|
|
|
Field name if the error is related to a specific field |
|
|
Specific code for the error situation, e.g. "FIELD_TO_LONG" |
|
|
Human readable description of the error code, e.g. "Field allows max 255 characters" |
2.4.2. Error codes
Error code | Description |
---|---|
|
An unexpected error occurred |
|
Validation of request failed |
|
Resource does not exist |
|
Resource already exists |
|
Provided offset id does not exist |
|
Server was not able to parse the request |
|
Provided media type is not supported, server supports application/json |
|
Server does not accept the request |
|
Request is unauthorized |
|
Device is not registered |
|
Provided session expiry time exceeds the maximum allowed value |
|
Too many concurrent active activation sessions |
|
Server failed to create activation code |
|
No active sessions found for the device |
|
Device is locked. See device API for details |
|
Device is not locked |
|
Provided session is not an activation session |
|
Authentication level is not allowed |
|
Authentication method is not configured for this app config |
|
Authentication method and level does not match each other |
|
Authentication method is not activated for the device |
|
Provided session is not an authentication session |
|
Provided session is not an offline authentication session |
|
The purpose of the provided session is not supported |
|
The application configuration provided is not valid. |
|
The application ID was not found. |
|
The APNS key is used by one or more application configurations |
|
The End-to-End key has status ENABLED and is considered in use |
|
The patch request is not of a valid format and cannot be mapped to the object that is supposed to be updated. |
|
Authentication request has sendPush flag set to true, but nativePushEnabled option in app configuration is false. |
|
The session has expired and can no longer be used |
|
The session has already had a failed or successful outcome |
|
The session is cancelled and can no longer be used |
|
The specified authentication method is locked. If the method in question is an offline-method, other methods might still work. |
|
The authentication method you are trying to unlock is not locked |
|
Authentication method is required in the request |
|
The request is missing one or more request parameters |
|
The purpose of the given session is not perform recovery. |
|
Too many device IDs were passed when listing sessions for devices; the number of devices is limited to 100. |
|
Failed to delete face authentication method of device due to Server Side Face Adapter error or unavailability. |
Example request
A request that triggers a validation error will produce a
422 Unprocessable Entity
response:
HTTP/1.1 422 Unprocessable Entity
X-Correlation-UUID: 15eb40a8-b0c7-4b66-95a6-6e12a1768911
Content-Type: application/json
Content-Length: 13970
{
"status" : 422,
"code" : "REST_VALIDATION_FAULT",
"message" : "Parameter validation failed on request /smart-device/v1/devices/0f7cd0a7-879f-49a6-92b8-6dab6abc94db/sessions. For more information see field specific errors.",
"correlation_id" : "15eb40a8-b0c7-4b66-95a6-6e12a1768911",
"errors" : [ {
"field" : "limit",
"code" : "REST_TYPE_MISMATCH",
"message" : "Failed to convert property value of type 'java.lang.String' to required type 'int' for property 'limit'; For input string: \"pp\""
}, {
"field" : "offset",
"code" : "REST_TYPE_MISMATCH",
"message" : "Failed to convert property value of type 'java.lang.String' to required type 'java.util.UUID' for property 'offset'; Invalid UUID string: ll"
} ],
"developer_message" : "Validation failed for argument [2] in org.springframework.http.ResponseEntity<com.encapsecurity.encap.rest.api.dto.PaginationResponseDTO<com.encapsecurity.encap.rest.api.dto.DeviceSessionDetailsDTO>> com.encapsecurity.encap.rest.controller.DeviceController.getSessionsForDevice(com.encapsecurity.encap.domain.EncapPrincipal,java.util.UUID,com.encapsecurity.encap.rest.api.dto.PaginationRequestDTO) with 2 errors: [Field error in object 'paginationRequestDTO' on field 'limit': rejected value [pp]; codes [typeMismatch.paginationRequestDTO.limit,typeMismatch.limit,typeMismatch.int,typeMismatch]; arguments [org.springframework.context.support.DefaultMessageSourceResolvable: codes [paginationRequestDTO.limit,limit]; arguments []; default message [limit]]; default message [Failed to convert property value of type 'java.lang.String' to required type 'int' for property 'limit'; For input string: \"pp\"]] [Field error in object 'paginationRequestDTO' on field 'offset': rejected value [ll]; codes [typeMismatch.paginationRequestDTO.offset,typeMismatch.offset,typeMismatch.java.util.UUID,typeMismatch]; arguments [org.springframework.context.support.DefaultMessageSourceResolvable: codes [paginationRequestDTO.offset,offset]; arguments []; default message [offset]]; default message [Failed to convert property value of type 'java.lang.String' to required type 'java.util.UUID' for property 'offset'; Invalid UUID string: ll]] \norg.springframework.web.bind.MethodArgumentNotValidException: Validation failed for argument [2] in org.springframework.http.ResponseEntity<com.encapsecurity.encap.rest.api.dto.PaginationResponseDTO<com.encapsecurity.encap.rest.api.dto.DeviceSessionDetailsDTO>> com.encapsecurity.encap.rest.controller.DeviceController.getSessionsForDevice(com.encapsecurity.encap.domain.EncapPrincipal,java.util.UUID,com.encapsecurity.encap.rest.api.dto.PaginationRequestDTO) with 2 errors: [Field error in object 'paginationRequestDTO' on field 'limit': rejected value [pp]; codes [typeMismatch.paginationRequestDTO.limit,typeMismatch.limit,typeMismatch.int,typeMismatch]; arguments [org.springframework.context.support.DefaultMessageSourceResolvable: codes [paginationRequestDTO.limit,limit]; arguments []; default message [limit]]; default message [Failed to convert property value of type 'java.lang.String' to required type 'int' for property 'limit'; For input string: \"pp\"]] [Field error in object 'paginationRequestDTO' on field 'offset': rejected value [ll]; codes [typeMismatch.paginationRequestDTO.offset,typeMismatch.offset,typeMismatch.java.util.UUID,typeMismatch]; arguments [org.springframework.context.support.DefaultMessageSourceResolvable: codes [paginationRequestDTO.offset,offset]; arguments []; default message [offset]]; default message [Failed to convert property value of type 'java.lang.String' to required type 'java.util.UUID' for property 'offset'; Invalid UUID string: ll]] \n\tat org.springframework.web.method.annotation.ModelAttributeMethodProcessor.resolveArgument(ModelAttributeMethodProcessor.java:158)\n\tat org.springframework.web.method.support.HandlerMethodArgumentResolverComposite.resolveArgument(HandlerMethodArgumentResolverComposite.java:122)\n\tat org.springframework.web.method.support.InvocableHandlerMethod.getMethodArgumentValues(InvocableHandlerMethod.java:224)\n\tat org.springframework.web.method.support.InvocableHandlerMethod.invokeForRequest(InvocableHandlerMethod.java:178)\n\tat org.springframework.web.servlet.mvc.method.annotation.ServletInvocableHandlerMethod.invokeAndHandle(ServletInvocableHandlerMethod.java:118)\n\tat org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerAdapter.invokeHandlerMethod(RequestMappingHandlerAdapter.java:926)\n\tat org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerAdapter.handleInternal(RequestMappingHandlerAdapter.java:831)\n\tat org.springframework.web.servlet.mvc.method.AbstractHandlerMethodAdapter.handle(AbstractHandlerMethodAdapter.java:87)\n\tat org.springframework.web.servlet.DispatcherServlet.doDispatch(DispatcherServlet.java:1089)\n\tat org.springframework.web.servlet.DispatcherServlet.doService(DispatcherServlet.java:979)\n\tat org.springframework.web.servlet.FrameworkServlet.processRequest(FrameworkServlet.java:1014)\n\tat org.springframework.web.servlet.FrameworkServlet.doGet(FrameworkServlet.java:903)\n\tat jakarta.servlet.http.HttpServlet.service(HttpServlet.java:633)\n\tat org.springframework.web.servlet.FrameworkServlet.service(FrameworkServlet.java:885)\n\tat org.springframework.test.web.servlet.TestDispatcherServlet.service(TestDispatcherServlet.java:72)\n\tat jakarta.servlet.http.HttpServlet.service(HttpServlet.java:723)\n\tat org.springframework.mock.web.MockFilterChain$ServletFilterProxy.doFilter(MockFilterChain.java:165)\n\tat org.springframework.mock.web.MockFilterChain.doFilter(MockFilterChain.java:132)\n\tat com.encapsecurity.encap.rest.filter.BasicAuthenticationFilter.doFilterInternal(BasicAuthenticationFilter.java:89)\n\tat org.springframework.web.filter.OncePerRequestFilter.doFilter(OncePerRequestFilter.java:116)\n\tat org.springframework.mock.web.MockFilterChain.doFilter(MockFilterChain.java:132)\n\tat com.encapsecurity.encap.rest.filter.RequestCorrelationIdFilter.doFilterInternal(RequestCorrelationIdFilter.java:22)\n\tat org.springframework.web.filter.OncePerRequestFilter.doFilter(OncePerRequestFilter.java:116)\n\tat org.springframework.mock.web.MockFilterChain.doFilter(MockFilterChain.java:132)\n\tat com.encapsecurity.encap.config.filter.AuditContextFilter.doFilter(AuditContextFilter.java:64)\n\tat org.springframework.mock.web.MockFilterChain.doFilter(MockFilterChain.java:132)\n\tat org.springframework.test.web.servlet.MockMvc.perform(MockMvc.java:201)\n\tat com.encapsecurity.encap.rest.controller.ExceptionThrowingControllerTest.typeMismatchOnPaginationRequestParam(ExceptionThrowingControllerTest.java:111)\n\tat java.base/jdk.internal.reflect.DirectMethodHandleAccessor.invoke(Unknown Source)\n\tat java.base/java.lang.reflect.Method.invoke(Unknown Source)\n\tat org.junit.platform.commons.util.ReflectionUtils.invokeMethod(ReflectionUtils.java:775)\n\tat org.junit.platform.commons.support.ReflectionSupport.invokeMethod(ReflectionSupport.java:479)\n\tat org.junit.jupiter.engine.execution.MethodInvocation.proceed(MethodInvocation.java:60)\n\tat org.junit.jupiter.engine.execution.InvocationInterceptorChain$ValidatingInvocation.proceed(InvocationInterceptorChain.java:131)\n\tat org.junit.jupiter.engine.extension.TimeoutExtension.intercept(TimeoutExtension.java:161)\n\tat org.junit.jupiter.engine.extension.TimeoutExtension.interceptTestableMethod(TimeoutExtension.java:152)\n\tat org.junit.jupiter.engine.extension.TimeoutExtension.interceptTestMethod(TimeoutExtension.java:91)\n\tat org.junit.jupiter.engine.execution.InterceptingExecutableInvoker$ReflectiveInterceptorCall.lambda$ofVoidMethod$0(InterceptingExecutableInvoker.java:112)\n\tat org.junit.jupiter.engine.execution.InterceptingExecutableInvoker.lambda$invoke$0(InterceptingExecutableInvoker.java:94)\n\tat org.junit.jupiter.engine.execution.InvocationInterceptorChain$InterceptedInvocation.proceed(InvocationInterceptorChain.java:106)\n\tat org.junit.jupiter.engine.execution.InvocationInterceptorChain.proceed(InvocationInterceptorChain.java:64)\n\tat org.junit.jupiter.engine.execution.InvocationInterceptorChain.chainAndInvoke(InvocationInterceptorChain.java:45)\n\tat org.junit.jupiter.engine.execution.InvocationInterceptorChain.invoke(InvocationInterceptorChain.java:37)\n\tat org.junit.jupiter.engine.execution.InterceptingExecutableInvoker.invoke(InterceptingExecutableInvoker.java:93)\n\tat org.junit.jupiter.engine.execution.InterceptingExecutableInvoker.invoke(InterceptingExecutableInvoker.java:87)\n\tat org.junit.jupiter.engine.descriptor.TestMethodTestDescriptor.lambda$invokeTestMethod$7(TestMethodTestDescriptor.java:216)\n\tat org.junit.platform.engine.support.hierarchical.ThrowableCollector.execute(ThrowableCollector.java:73)\n\tat org.junit.jupiter.engine.descriptor.TestMethodTestDescriptor.invokeTestMethod(TestMethodTestDescriptor.java:212)\n\tat org.junit.jupiter.engine.descriptor.TestMethodTestDescriptor.execute(TestMethodTestDescriptor.java:137)\n\tat org.junit.jupiter.engine.descriptor.TestMethodTestDescriptor.execute(TestMethodTestDescriptor.java:69)\n\tat org.junit.platform.engine.support.hierarchical.NodeTestTask.lambda$executeRecursively$6(NodeTestTask.java:156)\n\tat org.junit.platform.engine.support.hierarchical.ThrowableCollector.execute(ThrowableCollector.java:73)\n\tat org.junit.platform.engine.support.hierarchical.NodeTestTask.lambda$executeRecursively$8(NodeTestTask.java:146)\n\tat org.junit.platform.engine.support.hierarchical.Node.around(Node.java:137)\n\tat org.junit.platform.engine.support.hierarchical.NodeTestTask.lambda$executeRecursively$9(NodeTestTask.java:144)\n\tat org.junit.platform.engine.support.hierarchical.ThrowableCollector.execute(ThrowableCollector.java:73)\n\tat org.junit.platform.engine.support.hierarchical.NodeTestTask.executeRecursively(NodeTestTask.java:143)\n\tat org.junit.platform.engine.support.hierarchical.NodeTestTask.execute(NodeTestTask.java:100)\n\tat org.junit.platform.engine.support.hierarchical.ForkJoinPoolHierarchicalTestExecutorService$ExclusiveTask.exec(ForkJoinPoolHierarchicalTestExecutorService.java:274)\n\tat java.base/java.util.concurrent.ForkJoinTask.doExec(Unknown Source)\n\tat java.base/java.util.concurrent.ForkJoinPool$WorkQueue.tryRemoveAndExec(Unknown Source)\n\tat java.base/java.util.concurrent.ForkJoinTask.awaitDone(Unknown Source)\n\tat java.base/java.util.concurrent.ForkJoinTask.join(Unknown Source)\n\tat org.junit.platform.engine.support.hierarchical.ForkJoinPoolHierarchicalTestExecutorService.joinConcurrentTasksInReverseOrderToEnableWorkStealing(ForkJoinPoolHierarchicalTestExecutorService.java:201)\n\tat org.junit.platform.engine.support.hierarchical.ForkJoinPoolHierarchicalTestExecutorService.invokeAll(ForkJoinPoolHierarchicalTestExecutorService.java:167)\n\tat org.junit.platform.engine.support.hierarchical.NodeTestTask.lambda$executeRecursively$6(NodeTestTask.java:160)\n\tat org.junit.platform.engine.support.hierarchical.ThrowableCollector.execute(ThrowableCollector.java:73)\n\tat org.junit.platform.engine.support.hierarchical.NodeTestTask.lambda$executeRecursively$8(NodeTestTask.java:146)\n\tat org.junit.platform.engine.support.hierarchical.Node.around(Node.java:137)\n\tat org.junit.platform.engine.support.hierarchical.NodeTestTask.lambda$executeRecursively$9(NodeTestTask.java:144)\n\tat org.junit.platform.engine.support.hierarchical.ThrowableCollector.execute(ThrowableCollector.java:73)\n\tat org.junit.platform.engine.support.hierarchical.NodeTestTask.executeRecursively(NodeTestTask.java:143)\n\tat org.junit.platform.engine.support.hierarchical.NodeTestTask.execute(NodeTestTask.java:100)\n\tat org.junit.platform.engine.support.hierarchical.ForkJoinPoolHierarchicalTestExecutorService$ExclusiveTask.exec(ForkJoinPoolHierarchicalTestExecutorService.java:274)\n\tat java.base/java.util.concurrent.ForkJoinTask.doExec(Unknown Source)\n\tat java.base/java.util.concurrent.ForkJoinPool$WorkQueue.tryRemoveAndExec(Unknown Source)\n\tat java.base/java.util.concurrent.ForkJoinTask.awaitDone(Unknown Source)\n\tat java.base/java.util.concurrent.ForkJoinTask.join(Unknown Source)\n\tat org.junit.platform.engine.support.hierarchical.ForkJoinPoolHierarchicalTestExecutorService.joinConcurrentTasksInReverseOrderToEnableWorkStealing(ForkJoinPoolHierarchicalTestExecutorService.java:201)\n\tat org.junit.platform.engine.support.hierarchical.ForkJoinPoolHierarchicalTestExecutorService.invokeAll(ForkJoinPoolHierarchicalTestExecutorService.java:167)\n\tat org.junit.platform.engine.support.hierarchical.NodeTestTask.lambda$executeRecursively$6(NodeTestTask.java:160)\n\tat org.junit.platform.engine.support.hierarchical.ThrowableCollector.execute(ThrowableCollector.java:73)\n\tat org.junit.platform.engine.support.hierarchical.NodeTestTask.lambda$executeRecursively$8(NodeTestTask.java:146)\n\tat org.junit.platform.engine.support.hierarchical.Node.around(Node.java:137)\n\tat org.junit.platform.engine.support.hierarchical.NodeTestTask.lambda$executeRecursively$9(NodeTestTask.java:144)\n\tat org.junit.platform.engine.support.hierarchical.ThrowableCollector.execute(ThrowableCollector.java:73)\n\tat org.junit.platform.engine.support.hierarchical.NodeTestTask.executeRecursively(NodeTestTask.java:143)\n\tat org.junit.platform.engine.support.hierarchical.NodeTestTask.execute(NodeTestTask.java:100)\n\tat org.junit.platform.engine.support.hierarchical.ForkJoinPoolHierarchicalTestExecutorService$ExclusiveTask.exec(ForkJoinPoolHierarchicalTestExecutorService.java:274)\n\tat java.base/java.util.concurrent.ForkJoinTask.doExec(Unknown Source)\n\tat java.base/java.util.concurrent.ForkJoinPool$WorkQueue.topLevelExec(Unknown Source)\n\tat java.base/java.util.concurrent.ForkJoinPool.scan(Unknown Source)\n\tat java.base/java.util.concurrent.ForkJoinPool.runWorker(Unknown Source)\n\tat java.base/java.util.concurrent.ForkJoinWorkerThread.run(Unknown Source)\n"
}
2.5. Headers
2.5.1. Request header
Name | Description |
---|---|
|
Optional request header with a UUID for the request, if not present the server will generate a UUID. If the provided UUID is invalid server will respond with HTTP code 400 |
|
Authentication is performed via HTTP Basic Auth, and an API key is provided as the basic auth username value and the API secret as the password value, username and password is expected to be separated with a ':'. This header is required. |
2.5.2. Response header
Every response has the following header(s):
Name | Description |
---|---|
|
A UUID for the request. Id can be provided in the request header, if not provided it will be generated by the server |
2.6. Pagination
All Encap REST APIs that returns a collection of data supports pagination.
2.6.1. Request
Pagination requests has the following request parameters:
Parameter | Description |
---|---|
|
Id of the first session to include in response. Offset is optional, if not provided the result will start with the first session in the device history |
|
The number of objects on each page. Limit is optional, if not provided the defualt of 100 will be used. |
2.6.2. Response
Pagination response has the following structure:
Field | Type | Description |
---|---|---|
|
|
Holds the url to the previous set of items |
|
|
Holds the url to the next set of items |
|
|
Offset as passed in request |
|
|
The number of items on each page |
|
|
Items contains a list of the paginated objects for the request |
Example of pagination response:
HTTP/1.1 200 OK
X-Correlation-UUID: 7ef78cd4-74c5-44aa-8e87-eceaa4218062
Content-Type: application/json
Content-Length: 1541
{
"previous" : "/smart-device/v1/devices/92c478fc-82d1-48b2-8f91-75c830995d74/sessions?limit=3&offset=dff3245b-6385-47cb-90dd-37166f3ab38c",
"next" : "/smart-device/v1/devices/92c478fc-82d1-48b2-8f91-75c830995d74/sessions?limit=3&offset=e8839236-31bd-461d-af20-f05fe68be14d",
"offset" : "5045a14c-6ad3-415a-a079-b87916867a16",
"limit" : 3,
"items" : [ {
"session_id" : "3a30b6f6-64f6-42cd-80cc-fbd2bfbdbfdd",
"device_id" : "3826d15c-21a4-4d9c-9d48-3ce9ed43a88d",
"ref" : "/enrollments/3a30b6f6-64f6-42cd-80cc-fbd2bfbdbfdd",
"purpose" : "ACTIVATION",
"state" : "SUCCESS",
"client_only" : false,
"session_created_on" : "2025-03-17T16:58:08.097UTC",
"session_expires_on" : "2025-03-17T16:58:08.097UTC",
"offline" : false
}, {
"session_id" : "e93c4c18-23cc-461f-b66c-a04e65a91328",
"device_id" : "3826d15c-21a4-4d9c-9d48-3ce9ed43a88d",
"ref" : "/authentications/e93c4c18-23cc-461f-b66c-a04e65a91328",
"purpose" : "AUTHENTICATION",
"state" : "IN_PROGRESS",
"client_only" : false,
"session_created_on" : "2025-03-17T16:58:08.097UTC",
"session_expires_on" : "2025-03-17T16:58:08.097UTC",
"offline" : false
}, {
"session_id" : "f1140d34-9c21-4ff2-8d39-4cec8f84ef27",
"device_id" : "3826d15c-21a4-4d9c-9d48-3ce9ed43a88d",
"purpose" : "DELETE_RECOVERY",
"state" : "IN_PROGRESS",
"client_only" : false,
"session_created_on" : "2025-03-17T16:58:08.097UTC",
"session_expires_on" : "2025-03-17T16:58:08.097UTC",
"offline" : false
} ]
}
3. Smart Device API
3.1. Common objects
3.1.1. The risk attributes object
Field | Type | Description |
---|---|---|
|
|
The name of the risk attribute |
|
|
The value of the risk attribute |
Risk attributes
Encap can collect a set af risk attributes for each operation, what attributes to collect is configured in the application configuration
Risk attribute | Config attribute | Type | Description | Platform |
---|---|---|---|---|
|
|
String |
A fingerprint of the operating system. This can be used for detecting whether the device is running a custom ROM or operating system |
Android |
|
|
String |
The operating system version. Examples: 4.1.2, 6.0.1 |
iOS, Android |
|
|
String |
The input method that was used to enter text in the application. Can be used to detect when a custom keyboard is used on the device. For more info on input methods please see: https://developer.android.com/guide/topics/text/creating-input-method |
Android |
|
|
Boolean |
Indicate whether the application running can be debugged using a source level debugger, either by manifest entry or in emulator |
Android |
|
|
Boolean |
Indicate whether debug is enabled on device, either by user setting (USB-debugging enabled) or when running in emulator (debugging enabled by default) |
Android |
|
|
Boolean |
Indicate whether a debugger is connected to the application |
Android |
|
|
Boolean |
Indicate whether the application is running in an emulator |
Android |
|
|
Boolean |
Indicate whether the device has been rooted/jail-broken. For iOS this value has to be passed to Encap using the |
iOS, Android |
|
|
Boolean |
Indicate whether the device has secure screen enabled or not |
iOS, Android |
|
|
String |
The host address the request originated from. It will contain the value of X-Forwarded-For (XFF) header from the request and can contain multiple IP addresses depending on proxy and load balancers. If XFF is not present we will use the remote address of the request. It can be either the IP of the client or the last proxy that sent the request, it is specified by the value of the Common Gateway Interface (CGI) variable REMOTE_ADDR. |
iOS, Android |
|
|
Base64 |
SHA-256 hash of the public key of certificate of the application signer. If there are more signers, each hash is comma separated. This can be used for detecting whether the application has been re-signed. |
Android |
|
|
String |
HTTP User agent from smart device application. |
iOS, Android |
|
|
Base64 |
SHA256 hash of the unique hardware device ID of the client device. |
iOS, Android |
|
|
String |
The manufacturer of the device. Examples: "Apple", "Samsung". |
iOS, Android |
|
|
String |
The device’s model. Examples: "iPad2,2", "Nexus S". |
iOS, Android |
|
|
String |
Indicates the OperatingSystemType of the device, either Android or iOS. |
iOS, Android |
|
|
Base64 |
SHA256 hash of the application name. The server can use this for detecting re-packaging. |
iOS, Android |
|
|
JSON |
An array containing the client network interfaces. This includes the type of the network and the IP address, and the list can contain multiple interfaces if the user’s device is connected to more than one at the time of the transaction. Example: [{"Type":"Cellular","IPAddress":"123.123.123.123"},{"Type":"Wifi","IPAddress":"124.124.124.124"}] |
iOS, Android |
|
|
String |
The status of the hardware protected key signature preformed by the Encap client SDK. |
iOS, Android |
|
|
String |
The result of the hardware protected key signature verification on the Encap server. |
iOS, Android |
|
|
Integer |
Provide battery level of the device. |
iOS, Android |
|
|
Boolean |
Indicate whether the device is being charged/connected to a charger. |
iOS, Android |
|
|
Object |
Location data from the device. The response is part of the location object, and is not included as values in the risk attribute object. |
iOS, Android |
Hardware protected key server result
These are the possible values for the hw_key_server_result
attribute.
Status | Description | Validation strategy |
---|---|---|
SIGNATURE_VERIFICATION_SUCCESS |
Verification of the hardware protected key signature was successful |
Operation is successful in both strategy modes. |
SIGNATURE_VERIFICATION_FAILED |
Verification of the hardware protected key signature failed. |
Operation will fail in SUPPORTED mode. |
NOT_ACTIVATED_WITH_HW_KEY |
Signature could not be verified because registration was not activated with hardware protected keys. Only applicable for authentication. |
Operation will not fail regardless of strategy mode. |
NOT_PROVIDED_BY_CLIENT |
The client is on an Encap version that does not support the hardware protected key feature. Only applicable for enrollment. |
Operation will not fail regardless of strategy mode. The device will be activated without hardware protected keys, and the device will be able to perform all operations but will not be able to use the hardware protected key feature. |
Hardware protected key client statuses
These are the possible values for the hw_key_client_status
attribute.
Status | Description | Operation |
---|---|---|
OK_KEY_PROVIDED |
Activation of hardware protected keys was successful on the client. |
Enrollment |
OK_SIGNED_SUCCESS |
Authentication challenge was successfully signed with the hardware protected key on the client. |
Authentication |
INFO_NO_HARDWARE_SUPPORT |
The client device does not have Secure Enclave, or it is running in a simulator. Only for iOS |
Enrollment |
INFO_NO_OPERATING_SYSTEM_SUPPORT |
The client operating system does not support hardware backed keys. It is supported on:
|
Enrollment |
INFO_NOT_ACTIVATED_WITH_HW_KEY |
The registration was not activated with hardware protected keys support for one or more of the following reasons:
|
Authentication |
ERR_KEY_GENERATION_FAILED |
Unexpected error during generation of keypair on client. |
Enrollment |
ERR_RETRIEVE_PUBLIC_KEY_FAILED |
Unexpected error retrieving public key. Only on iOS. |
Enrollment |
ERR_RETRIEVE_PRIVATE_KEY_REF_FAILED |
Unexpected error when retrieving reference to private key. |
Enrollment and Authentication |
ERR_SIGN_OPERATION_FAILED |
Unexpected error when generating signature. |
Enrollment and Authentication |
3.1.2. The location object
Encap can collect the location of the device for each operation, given that it is enabled in the application configuration. This requires the end user to give the application permission to use the location of the device.
Field | Type | Description |
---|---|---|
|
|
Location accuracy |
|
|
Location altitude |
|
|
Location latitude |
|
|
Location longitude |
3.1.3. The operation context object
Field | Type | Description |
---|---|---|
|
|
Context title, that is base64 encoded twice. The double base64 encoding is a bug, the documentation will be updated once it’s fixed |
|
|
Context content, that is base64 encoded twice. The double base64 encoding is a bug, the documentation will be updated once it’s fixed |
|
|
The MIME type of the content |
3.1.4. The session status object
Field | Type | Description |
---|---|---|
|
|
The status of the session. See session status for details. |
|
|
A description of the session status |
|
|
The state of the session. See session state for details. |
Session statuses
Session Status | Description |
---|---|
|
Operation is in progress |
|
Operation is authenticated, but not complete |
|
Operation has completed successfully |
|
Operation failed because the session was cancelled by the device |
|
Operation failed because the session was cancelled by the service provider |
|
Operation failed because the session was cancelled by the device. The device started a deactivation of authentication method(s) |
|
Operation failed because the session was cancelled when a reactivation was started |
|
Operation failed because the session has expired |
|
Operation failed because the device is locked |
|
Operation failed because the device is locked by admin |
|
Operation failed because the device is locked by device verification |
|
Operation failed because the device is locked by pin verification |
|
Operation failed because the device is locked by incorrect salt key id |
|
Operation failed because the session callback failed. Legacy value from synchronous callback model. |
|
Operation failed because the device provided incorrect signed challenge |
|
Operation failed because the SafetyNet attestation validation failed |
|
Operation failed because the intermediate push attestation validation failed |
|
Operation failed because of missing intermediate push attestation details |
|
Operation failed because of missing SafetyNet attestation details |
|
Operation failed because authorization token validation failed |
|
Operation failed because the session was cancelled when recovery was started |
|
Operation failed because the recovery does not exist |
|
Operation failed because the recovery is locked |
|
Operation failed because the geofencing validation failed |
|
Operation failed because the App Attest validation failed |
|
Operation failed because of missing App Attest attestation details |
|
Operation failed because the Play Integrity validation failed |
|
Operation failed because of missing Play Integrity attestation details |
|
Operation failed unexpectedly |
|
Operation failed because Server Side Face adapter encountered an error |
|
Operation failed because Server Side Face verification failed |
|
Operation failed because a replay attempt was blocked by the Server Side Face adapter |
Session states
Session State | Description |
---|---|
|
Session is in progress |
|
Session has completed successfully |
|
Operation and session failed |
3.1.5. The attestation object
Field | Type | Description |
---|---|---|
|
|
Play Integrity attestation status for the device |
|
|
Date of when the Play Integrity attestation was performed |
|
|
SafetyNet attestation status for the device |
|
|
Date of when the SafetyNet attestation was performed |
|
|
App Attest attestation status for the device. Only for iOS devices |
|
|
Date of when the App Attest attestation was performed. Only for iOS devices |
App Attest statuses
App Attest status | Description |
---|---|
|
Attestation verification was successful |
|
Attestation failed because the Apple App Attest server was unavailable |
|
Attestation failed because App Attest is not supported on device |
|
Attestation failed because of an unexpected error; check the error message for details |
|
Attestation failed because the request took longer than the configured timeout |
|
Validation of the attestation object failed because the integrity check failed |
Play Integrity statuses
Google Play Integrity status | Description |
---|---|
|
Attestation verification was successful |
|
Attestation failed because the integrity check failed |
|
Attestation failed because the Google Play app is unrecognized |
|
Attestation failed because the Google Play app is unlicensed |
|
Attestation failed because of incorrect package name |
|
Attestation failed because of invalid JWS format |
|
Attestation failed because Google Play Integrity returned an API exception |
|
Attestation failed due to request taking longer than the configured timeout |
|
Attestation failed because of incorrect nonce |
|
Attestation failed because of missing JWS |
|
Attestation failed because of missing status returned from the client |
|
Attestation failed because the timestamp is not within the lifetime of the Encap server session |
|
Attestation failed due to client doesn’t have Google Play or the version is too old |
SafetyNet statuses
SafetyNet status | Description |
---|---|
|
Attestation verification was successful |
|
Attestation failed because the signature is invalid |
|
Attestation failed because the integrity check failed |
|
Attestation failed because of incorrect package name |
|
Attestation failed because of incorrect certificate digest |
|
Attestation failed because of invalid hostname |
|
Attestation failed because of invalid JWS format |
|
Attestation failed because SafetyNet returned an API exception |
|
Attestation failed due to request taking longer than the configured timeout |
|
Attestation failed due to missing API key |
|
Attestation failed because of incorrect nonce |
|
Attestation failed because of missing JWS |
|
Attestation failed because of missing status returned from the client |
|
Attestation failed because the timestamp is not within the lifetime of the session |
|
Attestation failed due to client doesn’t have Google Play or the version is too old |
3.1.6. The geofencing object
Field | Type | Description |
---|---|---|
|
|
Country code on ISO 3166-1 Alpha-2 format. |
|
|
Status of the geofencing client operation. |
|
|
Status of the geofencing server validation. |
Geofencing client statuses
Geofencing Client Status | Description |
---|---|
|
Country code obtained. |
|
Location not requested by app or user declined. |
|
Device did not obtain a location within configured accuracy before timeout. |
|
Location was mocked/faked. |
|
Geocoder not supported on device. |
|
Geocoder network or service not available. |
|
Unexpected geocoder error. |
|
Geocoder call did not finish within time limit. |
|
The reverse geocode request yielded an empty result for current location. |
3.1.7. The recovery object
Field | Type | Description |
---|---|---|
|
|
Identifier of a given recovery. |
|
|
The recovery method used to set up the recovery. |
|
|
The recovery status of a given recovery. |
|
|
Date for when a given recovery was has been created. |
|
|
Date for when a given recovery has been updated. |
|
|
Date for when a given recovery has been used. |
Recovery status
Recovery status | Description |
---|---|
|
Recovery has been set up for the device and is ready to be used. |
|
Recovery has been deactivated. |
|
Recovery has been used to recover the device. |
|
Recovery has been locked. |
Recovery method
Recovery method | Description |
---|---|
|
Recovery is based on cloud backup, and a recovery key locked with PIN |
|
Recovery is based on cloud backup, and a recovery key locked with Server Side Face authentication |
3.2. Constants
3.2.1. Authentication methods
Authentication method (Variant 1) | Authentication method (Variant 2) | Description |
---|---|---|
|
|
Device |
|
|
PIN |
|
|
FaceID for iOS |
|
|
Fingerprint for Android |
|
|
TouchID for iOS where the registered fingerprints at activation time cannot be updated |
|
|
Biometric prompt for Android |
|
|
Server Side Face authentication |
|
|
Device (offline authentication) |
|
|
PIN (offline authentication) |
|
|
TouchID for iOS (offline authentication) |
|
|
Biometric prompt for Android (offline authentication) |
|
|
Fingerprint for Android (offline authentication) |
|
|
FaceID for iOS (offline authentication) |
3.2.2. Authentication levels
Authentication level | Description |
---|---|
|
Authentication level for one factor |
|
Authentication level for two factors |
3.2.3. Lock reason
Lock reason | Description |
---|---|
|
Device is unlocked |
|
The verification of one of the authentication factors failed. Wrong device and/or a second-factor error (incorrect PIN code or biometrics) |
|
The device is locked by an administrative operation |
|
The verification of the device factor failed for an authentication |
|
The verification of the second authentication factor failed (incorrect PIN code or biometrics) |
|
The device provided an incorrect Salt-key ID during authentication |
|
The verification of the hardware-protected key failed (the key provided was incorrect) |
|
The device is locked due to a failed offline authentication, caused by OTP verification code mismatch |
|
The verification of the SafetyNet attestation failed |
|
The verification of the intermediate push attestation failed |
|
The verification of the App Attest attestation failed |
|
The verification of the Play Integrity attestation failed |
3.2.4. Session purpose
Purpose | Description |
---|---|
|
Activation/enrollment of new device. |
|
Authentication. |
|
Activating an additional auth method for an existing device. |
|
Deactivating an auth method for an existing device. |
|
Setting up recovery for an existing device. |
|
An existing recovery has been used to perform a recovery to a new device. |
|
An existing recovery has been deleted. |
|
A notification sent to the old device when performing a recovery. |
3.3. Enrollment
Enrollments are used to create a binding between a supported customer mobile device/application instance and the Encap server, which can then be used for subsequent secure mobile authentication operations.
When enrollment is started, the Encap server returns a unique activation code which must be conveyed to the customer and supplied to the mobile application instance along with any additional biometric or non-biometric authentication factors. The underlying Encap mobile authentication technology will then complete a complex cryptographic handshake between the application instance and the Encap server which results in a secure binding between the application instance and the service, completing the enrollment process.
It is the responsibility of the client to establish a suitably secure trust channel in order to convey the activation code to the customer. For example, this can be done by displaying the code QR format in a previously-authenticated web session, where it can be captured by the device camera, but ultimately any secure, trusted channel can be used.
The enrollment process is asynchronous – a start operation is used to commence enrollment which then proceeds in the background. Successful completion or failure of an ongoing enrollment process can then be detected either by polling additional API operations or via a client-supplied REST callback interface. These two styles are illustrated below:
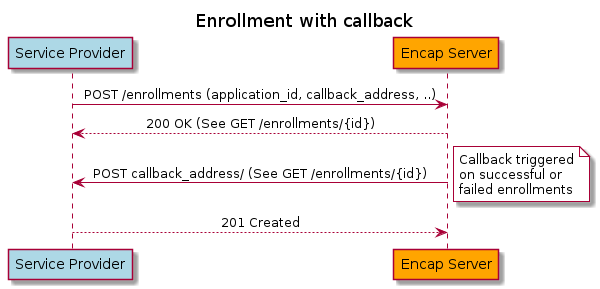
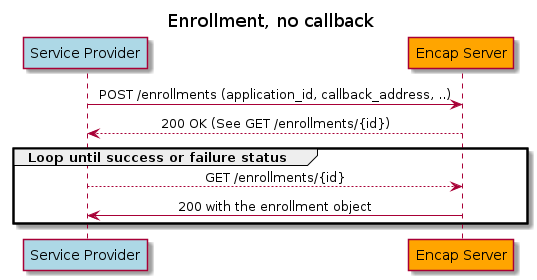
3.3.1. The enrollment object
Field | Type | Description |
---|---|---|
|
|
The UUID for the enrollment session |
|
|
The code to use for activation |
|
|
The application ID for the device |
|
|
The callback address for the device |
|
|
The UUID for the device |
|
|
The time for when the session was created. Returned in pattern yyyy-MM-dd’T’HH:mm:ss.SSS’UTC' |
|
|
The time for when the session will expire. Returned in pattern yyyy-MM-dd’T’HH:mm:ss.SSS’UTC' |
|
|
The authentication level of the enrollment. See authentication levels for options |
|
|
List of activated authentication methods (Variant 2). See authentication methods for possible values |
|
|
The push address for the device, this address is used when sending push to the device using FCM or APNS. |
|
|
A custom payload send to the device in the push notification. |
|
|
Explicit setting for the device to lock/unlock recovery. |
|
|
Operation context object that contains details to be shown after a successful the authentication. See operation_context object for details. |
|
|
Attestation object that contains details for all the attestations performed for the device. See attestation object for details. |
|
|
List that contains name and value for all risk attributes. See risk_attributes object for details. |
|
|
Status object that contains status details of the session. See session_status object for details. |
|
|
Location object that contains location details, see location object for details. |
|
|
Geofencing object that contains details of device location and geofencing validation. See geofencing object for details. |
3.3.2. Start enrollment of device
POST /api/smart-device/v1/enrollments
To start an enrollment of a device you create an enrollment object
Arguments
Path | Type | Required | Description |
---|---|---|---|
|
|
Yes |
The application id for the configuration to use during the enrollment |
|
|
No |
The authentication level for the operation. See authentication levels for options. If not provided default level TWO_FACTOR will be used |
|
|
No |
The callback address for the device |
|
|
No |
Life time in ms for for the session before it expires |
|
|
No |
A custom payload send to the device in the push notification, the payload can maximum contain 256 bytes and all characters must be in the Basic Multilingual Plane. Please note that the payload is sent in plain text and that the push channel is not a secure channel, to send data to the device over a secure channel use the post/pre operation context. |
|
|
No |
Allows to override recovery setting from app config and disable recovery during REST enrollment. |
|
|
No |
Operation context object that contains details to be shown after a successful the authentication. See operation_context object for details. |
Example request body
{
"application_id" : "encap",
"session_expiry_time" : 3000,
"callback_address" : "http://callback.url.com",
"post_operation_context" : {
"context_title_b64" : "VG1salpWUnBkR3hs",
"context_content_b64" : "VG1salpWUnBkR3hs",
"context_mime" : "text/plain"
},
"authentication_level" : "ONE_FACTOR",
"custom_push_payload" : "ServerA",
"recovery_lock" : false
}
Returns
Returns an enrollment object if the enrollment process is successfully initiated. Returns an error object if something goes wrong.
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/enrollments' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X POST \
-H 'Content-Type: application/json' \
-d '{
"application_id" : "encap",
"session_expiry_time" : 3000,
"callback_address" : "http://callback.url.com",
"post_operation_context" : {
"context_title_b64" : "VG1salpWUnBkR3hs",
"context_content_b64" : "VG1salpWUnBkR3hs",
"context_mime" : "text/plain"
},
"authentication_level" : "ONE_FACTOR",
"custom_push_payload" : "ServerA",
"recovery_lock" : false
}'
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 8fc1db7c-c345-44bc-b550-34f80e130324
Content-Type: application/json
Content-Length: 1809
{
"id" : "59cd11f7-2c1c-4ec1-be3d-93c919af7ebc",
"application_id" : "encap",
"activation_code" : "123456",
"callback_address" : "callback",
"device_id" : "5e966565-008c-4768-8ff7-faf997ef75f7",
"session_created_time" : "2025-03-17T16:58:10.404UTC",
"session_expiry_time" : "2025-03-17T18:58:10.404UTC",
"authentication_level" : "TWO_FACTOR",
"activated_authentication_methods" : [ "DEVICE", "DEVICE:PIN" ],
"location" : {
"accuracy" : "0.564",
"altitude" : "76.2352.3563",
"latitude" : "12.34234.143",
"longitude" : "32.24314.1431"
},
"post_operation_context" : {
"context_title_b64" : "VkdsMGJHVT0=",
"context_content_b64" : "UTI5dWRHVnVkQT09",
"context_mime" : "MIME"
},
"risk_attributes" : [ {
"name" : "is_debuggable",
"value" : "true"
}, {
"name" : "is_debugger_connected",
"value" : "true"
}, {
"name" : "input_method",
"value" : "input"
}, {
"name" : "is_emulator",
"value" : "true"
}, {
"name" : "operating_system_version",
"value" : "2.3"
}, {
"name" : "client_side_ip",
"value" : "[\"192.168.0.1\"]"
} ],
"session_status" : {
"status" : "IN_PROGRESS",
"description" : "Operation is in progress",
"state" : "IN_PROGRESS"
},
"attestation" : {
"safety_net_status" : "VERIFIED",
"safety_net_date" : "2025-03-17T16:58:10.404UTC",
"app_attest_status" : "VERIFIED",
"app_attest_date" : "2025-03-17T16:58:10.404UTC",
"play_integrity_status" : "VERIFIED",
"play_integrity_date" : "2025-03-17T16:58:10.404UTC"
},
"geofencing" : {
"country_code" : "NO",
"client_status" : "OK",
"server_boundary_validation" : "SUCCESS"
},
"push_address" : "123409238402394f3413r2e2f",
"custom_push_payload" : "ServerA",
"recovery_lock" : false
}
3.3.3. Get enrollment details
GET /api/smart-device/v1/enrollments/{id}
Getting the enrollment details lets you know the state of the enrollment and if finished it gives you access to risk data and all other attributes created as part of the enrollment process.
Returns
Returns an enrollment object if a valid identifier was provided, and returns an error otherwise.
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/enrollments/59cd11f7-2c1c-4ec1-be3d-93c919af7ebc' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X GET
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 68a8d4a2-75dd-412a-a18a-ed63c275b2c4
Content-Type: application/json
Content-Length: 1809
{
"id" : "59cd11f7-2c1c-4ec1-be3d-93c919af7ebc",
"application_id" : "encap",
"activation_code" : "123456",
"callback_address" : "callback",
"device_id" : "d648ada5-b75c-4c7c-ac46-02f3732cb131",
"session_created_time" : "2025-03-17T16:58:10.642UTC",
"session_expiry_time" : "2025-03-17T18:58:10.642UTC",
"authentication_level" : "TWO_FACTOR",
"activated_authentication_methods" : [ "DEVICE", "DEVICE:PIN" ],
"location" : {
"accuracy" : "0.564",
"altitude" : "76.2352.3563",
"latitude" : "12.34234.143",
"longitude" : "32.24314.1431"
},
"post_operation_context" : {
"context_title_b64" : "VkdsMGJHVT0=",
"context_content_b64" : "UTI5dWRHVnVkQT09",
"context_mime" : "MIME"
},
"risk_attributes" : [ {
"name" : "is_debuggable",
"value" : "true"
}, {
"name" : "is_debugger_connected",
"value" : "true"
}, {
"name" : "input_method",
"value" : "input"
}, {
"name" : "is_emulator",
"value" : "true"
}, {
"name" : "operating_system_version",
"value" : "2.3"
}, {
"name" : "client_side_ip",
"value" : "[\"192.168.0.1\"]"
} ],
"session_status" : {
"status" : "IN_PROGRESS",
"description" : "Operation is in progress",
"state" : "IN_PROGRESS"
},
"attestation" : {
"safety_net_status" : "VERIFIED",
"safety_net_date" : "2025-03-17T16:58:10.642UTC",
"app_attest_status" : "VERIFIED",
"app_attest_date" : "2025-03-17T16:58:10.642UTC",
"play_integrity_status" : "VERIFIED",
"play_integrity_date" : "2025-03-17T16:58:10.642UTC"
},
"geofencing" : {
"country_code" : "NO",
"client_status" : "OK",
"server_boundary_validation" : "SUCCESS"
},
"push_address" : "123409238402394f3413r2e2f",
"custom_push_payload" : "ServerA",
"recovery_lock" : false
}
3.3.4. Cancel enrollment
DELETE /api/smart-device/v1/enrollments/{sessionId}
To cancel an enrollment in progress thereby preventing the device from finishing the enrollment process
Returns
Returns 204 No Content if a valid identifier was provided and the process was canceled. Returns an error object if something goes wrong.
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/enrollments/59cd11f7-2c1c-4ec1-be3d-93c919af7ebc' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X DELETE
Example response
HTTP/1.1 204 No Content
X-Correlation-UUID: 9665f7df-3a3b-4d4a-b85a-c0941d007565
3.3.5. Callback
If ´callback_address´ is set, this will be a POST to the Service Provider’s ´callback_address´ with an enrollment object identical to the object that the Service Provider would get if get enrollment details was called.
See callback implementation and callback usage for more details.
Example request
POST /api/callback/v1/enroll HTTP/1.1
Content-Type: application/json
Content-Length: 1321
Host: localhost:8080
{
"id" : "fc5433e0-539c-4cdd-a44e-8e6b99daa5e9",
"application_id" : "encap",
"activation_code" : "123456",
"callback_address" : "http://callback.url.com",
"device_id" : "75120a9e-15d3-465c-8dd0-8c784f7f74b4",
"session_created_time" : "2025-03-17T16:58:10.175UTC",
"session_expiry_time" : "2025-03-17T16:58:10.175UTC",
"authentication_level" : "TWO_FACTOR",
"activated_authentication_methods" : [ "DEVICE", "DEVICE:PIN" ],
"location" : {
"accuracy" : "0.564",
"altitude" : "76.2352.3563",
"latitude" : "12.34234.143",
"longitude" : "32.24314.1431"
},
"post_operation_context" : {
"context_title_b64" : "VG1salpWUnBkR3hs",
"context_content_b64" : "VG1salpWUnBkR3hs",
"context_mime" : "text/plain"
},
"risk_attributes" : [ {
"name" : "is_debuggable",
"value" : "true"
}, {
"name" : "is_debugger_connected",
"value" : "true"
}, {
"name" : "input_method",
"value" : "input"
}, {
"name" : "is_emulator",
"value" : "true"
}, {
"name" : "operating_system_version",
"value" : "2.3"
}, {
"name" : "client_side_ip",
"value" : "[\"192.168.0.1\"]"
} ],
"session_status" : {
"status" : "SUCCESS",
"description" : "Operation has completed successfully",
"state" : "SUCCESS"
},
"recovery_lock" : false
}
Example response
HTTP/1.1 200 OK
3.3.6. Re-enrollment
Re-enrollment enables users to be re-enrolled and create new credentials on the same device ID given that they are still using the same device as they were originally enrolled on. This will remove complexity from Service Providers allowing them to reuse the device ID instead of creating a new one.
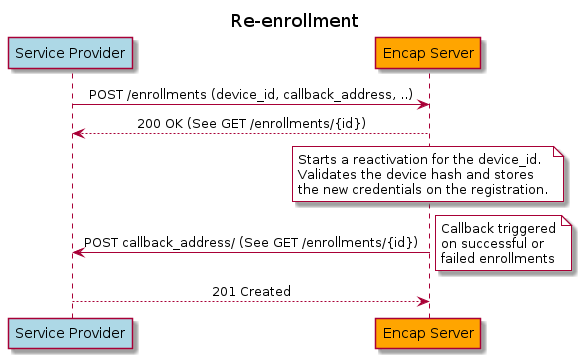
Limitations
-
Re-enrollment does not work on registrations that has been deactivated either by the Service Provider or by the end-user. In these scenarios the user needs to start a new enrollment.
-
End-user must be on the same device. Encap validates the device hash during the re-enrollment to confirm that the end-user is still using the same device. Generally, the device hash will remain unchanged after a re-installation of the app, but we have identified the following exceptions:
-
Android:
-
Factory reset may change the device hash.
-
Reinstalling the app after an upgrade from Android version 7 or below to 8 or above will cause changes to the device hash.
-
The device hash will not be carried over to a new device.
-
-
iOS:
-
Reinstalling the app from a non-encrypted backup after a factory reset
-
The device hash will not be carried over to a new device.
-
-
Start re-enrollment of device
POST /api/smart-device/v1/enrollments
To start a re-enrollment of a device you create an enrollment object that contains device_id
parameter.
application_id
is optional in this case.
Arguments
Path | Type | Required | Description |
---|---|---|---|
|
|
Yes |
The device id for the configuration to use during the re-enrollment |
|
|
No |
The application id for the configuration to use during the enrollment. If not provided we will use application id of the old registration.It is not allowed to change application id in e re-enrollment, if application id is provided it has to match the application id of the old registration. |
|
|
No |
The authentication level for the operation. See authentication levels for options. If not provided default level TWO_FACTOR will be used |
|
|
No |
The callback address for the device |
|
|
No |
Life time in ms for for the session before it expires |
|
|
No |
A custom payload send to the device in the push notification, the payload can maximum contain 256 bytes and all characters must be in the Basic Multilingual Plane. Please note that the payload is sent in plain text and that the push channel is not a secure channel, to send data to the device over a secure channel use the post/pre operation context. |
|
|
No |
Operation context object that contains details to be shown after a successful the authentication. See operation_context object for details. |
Example request body
{
"application_id" : "encap",
"session_expiry_time" : 3000,
"callback_address" : "http://callback.url.com",
"post_operation_context" : {
"context_title_b64" : "VG1salpWUnBkR3hs",
"context_content_b64" : "VG1salpWUnBkR3hs",
"context_mime" : "text/plain"
},
"authentication_level" : "ONE_FACTOR",
"custom_push_payload" : "ServerA",
"device_id" : "5b66d14b-7e36-486c-b6ce-3a9f5842c7ab"
}
Returns
Returns an enrollment object if the enrollment process is successfully initiated. Returns an error object if something goes wrong.
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/enrollments' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X POST \
-H 'Content-Type: application/json' \
-d '{
"application_id" : "encap",
"session_expiry_time" : 3000,
"callback_address" : "http://callback.url.com",
"post_operation_context" : {
"context_title_b64" : "VG1salpWUnBkR3hs",
"context_content_b64" : "VG1salpWUnBkR3hs",
"context_mime" : "text/plain"
},
"authentication_level" : "ONE_FACTOR",
"custom_push_payload" : "ServerA",
"device_id" : "5b66d14b-7e36-486c-b6ce-3a9f5842c7ab"
}'
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 0e02fc10-2cdc-4aa5-97db-43387a2bd0c9
Content-Type: application/json
Content-Length: 1809
{
"id" : "59cd11f7-2c1c-4ec1-be3d-93c919af7ebc",
"application_id" : "encap",
"activation_code" : "123456",
"callback_address" : "callback",
"device_id" : "020e8b85-a59c-419f-9a9c-fb078110e6ff",
"session_created_time" : "2025-03-17T16:58:11.127UTC",
"session_expiry_time" : "2025-03-17T18:58:11.127UTC",
"authentication_level" : "TWO_FACTOR",
"activated_authentication_methods" : [ "DEVICE", "DEVICE:PIN" ],
"location" : {
"accuracy" : "0.564",
"altitude" : "76.2352.3563",
"latitude" : "12.34234.143",
"longitude" : "32.24314.1431"
},
"post_operation_context" : {
"context_title_b64" : "VkdsMGJHVT0=",
"context_content_b64" : "UTI5dWRHVnVkQT09",
"context_mime" : "MIME"
},
"risk_attributes" : [ {
"name" : "is_debuggable",
"value" : "true"
}, {
"name" : "is_debugger_connected",
"value" : "true"
}, {
"name" : "input_method",
"value" : "input"
}, {
"name" : "is_emulator",
"value" : "true"
}, {
"name" : "operating_system_version",
"value" : "2.3"
}, {
"name" : "client_side_ip",
"value" : "[\"192.168.0.1\"]"
} ],
"session_status" : {
"status" : "IN_PROGRESS",
"description" : "Operation is in progress",
"state" : "IN_PROGRESS"
},
"attestation" : {
"safety_net_status" : "VERIFIED",
"safety_net_date" : "2025-03-17T16:58:11.127UTC",
"app_attest_status" : "VERIFIED",
"app_attest_date" : "2025-03-17T16:58:11.127UTC",
"play_integrity_status" : "VERIFIED",
"play_integrity_date" : "2025-03-17T16:58:11.127UTC"
},
"geofencing" : {
"country_code" : "NO",
"client_status" : "OK",
"server_boundary_validation" : "SUCCESS"
},
"push_address" : "123409238402394f3413r2e2f",
"custom_push_payload" : "ServerA",
"recovery_lock" : false
}
3.4. Authentication
Authentication operations are used to authenticate customers via previously-enrolled device/application instances. The authentication process is fully contextual, with context information supplied via the API that can be displayed to the customer before and/or after authentication has completed.
The authentication process is asynchronous – a start operation is used to commence an authentication process which then proceeds in the background. Successful completion or failure of an ongoing authentication process can then be detected either by polling via additional API requests or via a client-implemented REST callback interface. These two styles are illustrated below:
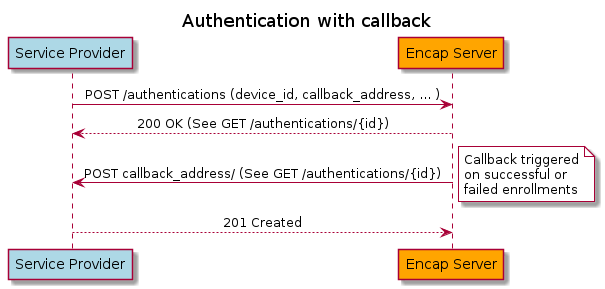
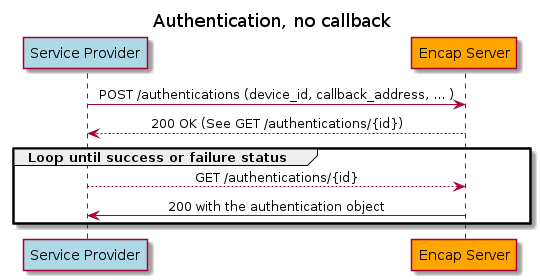
3.4.1. The authentication object
Field | Type | Description |
---|---|---|
|
|
The UUID for the authentication session |
|
|
The authentication level of the enrollment. See authentication levels for options |
|
|
The authentication method for the operation (Variant 1). See authentication methods for possible values |
|
|
The callback address for the device |
|
|
The UUID for the device |
|
|
Boolean for if a push has been sent or not |
|
|
The time for when the session was created. Returned in pattern yyyy-MM-dd’T’HH:mm:ss.SSS’UTC' |
|
|
The time for when the session will expire. Returned in pattern yyyy-MM-dd’T’HH:mm:ss.SSS’UTC' |
|
|
The purpose of the session. See session purpose for details. |
|
|
The base64 encoded client data used by the client in identify. |
|
|
A custom payload send to the device in the push notification. |
|
|
Operation context object that contains details to be shown before the authentication.See operation_context object for details. |
|
|
Operation context object that contains details to be shown after a successful the authentication. See operation_context object for details. |
|
|
List that contains name and value for all risk attributes. See risk_attributes object for details. |
|
|
Location object that contains location details, see location object for details. |
|
|
Status object that contains status details of the session. See session_status object for details. |
|
|
Attestation object that contains details for all the attestations performed for the device. See attestation object for details. |
|
|
Geofencing object that contains details of device location and geofencing validation. See geofencing object for details. |
3.4.2. Start authentication
POST /api/smart-device/v1/authentications
To start an authentication you create an authentication object
Arguments
Path | Type | Required | Description |
---|---|---|---|
|
|
Yes |
The UUID for the device |
|
|
No |
The authentication level for the operation. See authentication levels for options. If not provided default level TWO_FACTOR will be used |
|
|
No |
The authentication method for the operation (Variant 1). See authentication methods for possible values |
|
|
No |
The callback address for the device |
|
|
No |
Life time in ms for for the session before it expires |
|
|
No |
A custom payload send to the device in the push notification, the payload can maximum contain 256 bytes and all characters must be in the Basic Multilingual Plane. Please note that the payload is sent in plain text and that the push channel is not a secure channel, to send data to the device over a secure channel use the post/pre operation context. |
|
|
No |
Choose to send push or not. It requires that push notifications are allowed for the given appId. Default is true. |
|
|
No |
Operation context object that contains details to be shown before the authentication.See operation_context object for details. |
|
|
No |
Operation context object that contains details to be shown after a successful the authentication. See operation_context object for details. |
Example request body
{
"device_id" : "6a088f45-b28c-4d2e-8fe3-32ac6e3a99d3",
"authentication_level" : "TWO_FACTOR",
"authentication_method" : "DEVICE_PIN",
"callback_address" : "http://callback.url.com",
"session_expiry_time" : 30000,
"pre_operation_context" : {
"context_title_b64" : "VUhKbFZHbDBiR1U9",
"context_content_b64" : "VUhKbFEyOXVkR1Z1ZEE9PQ==",
"context_mime" : "text/plain"
},
"post_operation_context" : {
"context_title_b64" : "VUc5emRGUnBkR3hs",
"context_content_b64" : "VUc5emRFTnZiblJsYm5RPQ==",
"context_mime" : "text/plain"
},
"send_push" : true,
"custom_push_payload" : "ServerA"
}
Returns
Returns an authentication object if authentication process is successfully initiated. Returns an error object if something goes wrong.
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/authentications' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X POST \
-H 'Content-Type: application/json' \
-d '{
"device_id" : "6a088f45-b28c-4d2e-8fe3-32ac6e3a99d3",
"authentication_level" : "TWO_FACTOR",
"authentication_method" : "DEVICE_PIN",
"callback_address" : "http://callback.url.com",
"session_expiry_time" : 30000,
"pre_operation_context" : {
"context_title_b64" : "VUhKbFZHbDBiR1U9",
"context_content_b64" : "VUhKbFEyOXVkR1Z1ZEE9PQ==",
"context_mime" : "text/plain"
},
"post_operation_context" : {
"context_title_b64" : "VUc5emRGUnBkR3hs",
"context_content_b64" : "VUc5emRFTnZiblJsYm5RPQ==",
"context_mime" : "text/plain"
},
"send_push" : true,
"custom_push_payload" : "ServerA"
}'
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: d2dbd498-48c5-4a98-9c7c-4edd3b59cc44
Content-Type: application/json
Content-Length: 1916
{
"id" : "7a3069b6-d7e0-4247-8c9e-385ba9779644",
"authentication_level" : "TWO_FACTOR",
"authentication_method" : "DEVICE_PIN",
"callback_address" : "callback",
"device_id" : "bbabd88f-aec0-4af2-8333-d745babaf0af",
"push_sent" : true,
"custom_push_payload" : "ServerA",
"session_created_time" : "2025-03-17T16:58:08.671UTC",
"session_expiry_time" : "2025-03-17T18:58:08.671UTC",
"pre_operation_context" : {
"context_title_b64" : "VUhKbFZHbDBiR1U9",
"context_content_b64" : "VUhKbFEyOXVkR1Z1ZEE9PQ==",
"context_mime" : "MIME"
},
"post_operation_context" : {
"context_title_b64" : "VUc5emRGUnBkR3hs",
"context_content_b64" : "VUc5emRFTnZiblJsYm5RPQ==",
"context_mime" : "MIME"
},
"location" : {
"accuracy" : "0.564",
"altitude" : "76.2352.3563",
"latitude" : "12.34234.143",
"longitude" : "32.24314.1431"
},
"session_status" : {
"status" : "IN_PROGRESS",
"description" : "Operation is in progress",
"state" : "IN_PROGRESS"
},
"risk_attributes" : [ {
"name" : "is_debuggable",
"value" : "true"
}, {
"name" : "is_debugger_connected",
"value" : "true"
}, {
"name" : "input_method",
"value" : "input"
}, {
"name" : "is_emulator",
"value" : "true"
}, {
"name" : "operating_system_version",
"value" : "2.3"
}, {
"name" : "client_side_ip",
"value" : "[\"192.168.0.1\"]"
} ],
"client_data" : "YWJjZGVmMTIzNDU2",
"attestation" : {
"safety_net_status" : "VERIFIED",
"safety_net_date" : "2025-03-17T16:58:08.669UTC",
"app_attest_status" : "VERIFIED",
"app_attest_date" : "2025-03-17T16:58:08.669UTC",
"play_integrity_status" : "VERIFIED",
"play_integrity_date" : "2025-03-17T16:58:08.669UTC"
},
"geofencing" : {
"country_code" : "NO",
"client_status" : "OK",
"server_boundary_validation" : "SUCCESS"
},
"purpose" : "AUTHENTICATION"
}
3.4.3. Get authentication details
GET /api/smart-device/v1/authentications/{id}
Getting the authentication details lets you know the state of the authentication and if finished it gives you access to risk data and all other attributes created as part of the authentication process.
Returns
Returns an authentication object if a valid identifier was provided, and returns an error otherwise.
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/authentications/7a3069b6-d7e0-4247-8c9e-385ba9779644' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X GET
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 4a7413cc-816e-4e70-bba2-12f341ca9ed7
Content-Type: application/json
Content-Length: 1916
{
"id" : "7a3069b6-d7e0-4247-8c9e-385ba9779644",
"authentication_level" : "TWO_FACTOR",
"authentication_method" : "DEVICE_PIN",
"callback_address" : "callback",
"device_id" : "3e7a3692-9b9b-4cd2-a8ef-116a6d37b1aa",
"push_sent" : true,
"custom_push_payload" : "ServerA",
"session_created_time" : "2025-03-17T16:58:09.172UTC",
"session_expiry_time" : "2025-03-17T18:58:09.172UTC",
"pre_operation_context" : {
"context_title_b64" : "VUhKbFZHbDBiR1U9",
"context_content_b64" : "VUhKbFEyOXVkR1Z1ZEE9PQ==",
"context_mime" : "MIME"
},
"post_operation_context" : {
"context_title_b64" : "VUc5emRGUnBkR3hs",
"context_content_b64" : "VUc5emRFTnZiblJsYm5RPQ==",
"context_mime" : "MIME"
},
"location" : {
"accuracy" : "0.564",
"altitude" : "76.2352.3563",
"latitude" : "12.34234.143",
"longitude" : "32.24314.1431"
},
"session_status" : {
"status" : "IN_PROGRESS",
"description" : "Operation is in progress",
"state" : "IN_PROGRESS"
},
"risk_attributes" : [ {
"name" : "is_debuggable",
"value" : "true"
}, {
"name" : "is_debugger_connected",
"value" : "true"
}, {
"name" : "input_method",
"value" : "input"
}, {
"name" : "is_emulator",
"value" : "true"
}, {
"name" : "operating_system_version",
"value" : "2.3"
}, {
"name" : "client_side_ip",
"value" : "[\"192.168.0.1\"]"
} ],
"client_data" : "YWJjZGVmMTIzNDU2",
"attestation" : {
"safety_net_status" : "VERIFIED",
"safety_net_date" : "2025-03-17T16:58:09.170UTC",
"app_attest_status" : "VERIFIED",
"app_attest_date" : "2025-03-17T16:58:09.170UTC",
"play_integrity_status" : "VERIFIED",
"play_integrity_date" : "2025-03-17T16:58:09.170UTC"
},
"geofencing" : {
"country_code" : "NO",
"client_status" : "OK",
"server_boundary_validation" : "SUCCESS"
},
"purpose" : "AUTHENTICATION"
}
3.4.4. Cancel authentication
DELETE /api/smart-device/v1/authentications/{sessionId}
To cancel an authentication in progress thereby preventing the device from finishing the authentication process
Returns
Returns 204 No Content if a valid identifier was provided and the process was canceled. Returns an error object if something goes wrong.
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/authentications/7a3069b6-d7e0-4247-8c9e-385ba9779644' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X DELETE
Example response
HTTP/1.1 204 No Content
X-Correlation-UUID: 1cfbc255-3b98-44d0-b810-5cd27222fbdb
3.4.5. Callback
If ´callback_address´ is set, this will be a POST to the Service Provider’s ´callback_address´ with an authentication object identical to the object that the Service Provider would get if get authentication details was called.
See callback implementation and callback usage for more details.
Example request
POST /api/callback/v1/auth HTTP/1.1
Content-Type: application/json
Content-Length: 1409
Host: localhost:8080
{
"id" : "933b4729-3cca-402c-af9a-47815c84b27e",
"authentication_level" : "TWO_FACTOR",
"authentication_method" : "DEVICE_PIN",
"callback_address" : "http://callback.url.com",
"device_id" : "0c8c058b-35e8-4e10-8d12-600cc7df89f8",
"push_sent" : false,
"session_created_time" : "2025-03-17T16:58:06.640UTC",
"session_expiry_time" : "2025-03-17T16:58:06.640UTC",
"pre_operation_context" : {
"context_title_b64" : "VUhKbFZHbDBiR1U9",
"context_content_b64" : "VUhKbFEyOXVkR1Z1ZEE9PQ==",
"context_mime" : "text/plain"
},
"post_operation_context" : {
"context_title_b64" : "VUc5emRGUnBkR3hs",
"context_content_b64" : "VUc5emRFTnZiblJsYm5RPQ==",
"context_mime" : "text/plain"
},
"location" : {
"accuracy" : "0.564",
"altitude" : "76.2352.3563",
"latitude" : "12.34234.143",
"longitude" : "32.24314.1431"
},
"session_status" : {
"status" : "SUCCESS",
"description" : "Operation has completed successfully",
"state" : "SUCCESS"
},
"risk_attributes" : [ {
"name" : "is_debuggable",
"value" : "true"
}, {
"name" : "is_debugger_connected",
"value" : "true"
}, {
"name" : "input_method",
"value" : "input"
}, {
"name" : "is_emulator",
"value" : "true"
}, {
"name" : "operating_system_version",
"value" : "2.3"
}, {
"name" : "client_side_ip",
"value" : "[\"192.168.0.1\"]"
} ]
}
Example response
HTTP/1.1 200 OK
3.5. Offline Authentication
An API for doing offline authentications. Offline meaning the device does not have access to the internet. Data is transferred as a verification string to the device (for instance through a QR-code), and verified by a one time password
The offline flow runs as follows:
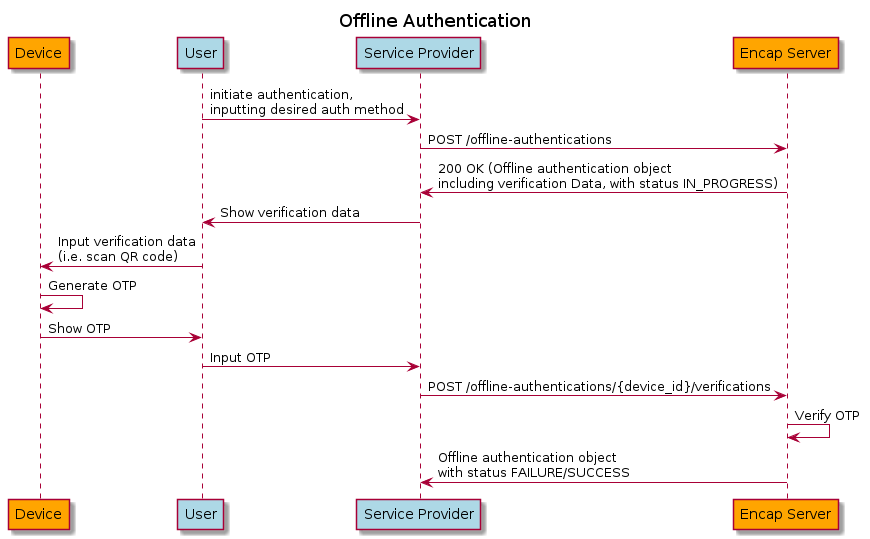
3.5.1. The offline authentication object
Field | Type | Description |
---|---|---|
|
|
The UUID of the authentication session |
|
|
The context used in the authentication |
|
|
String of data transferable to the client to complete the authentication |
|
|
The UUID for the device |
|
|
The time for when the session was created. Returned in pattern yyyy-MM-dd’T’HH:mm:ss.SSS’UTC' |
|
|
The time for when the session will expire. Returned in pattern yyyy-MM-dd’T’HH:mm:ss.SSS’UTC' |
|
|
The authentication method for the operation (Variant 1). See authentication methods for possible values |
|
|
The authentication level of the enrollment. See authentication levels for options |
|
|
Status object that contains status details of the session. See session_status object for details. |
3.5.2. Begin offline authentication
POST /api/smart-device/v1/offline-authentications
To start an offline authentication, you create an offline authentication object
Arguments
Path | Type | Required | Description |
---|---|---|---|
|
|
Yes |
The UUID of the device you want to authenticate |
|
|
No |
Amount of time until the authentication will expire in milliseconds |
|
|
No |
Authentication method you want to use (Variant 1). See authentication methods for possible values |
|
|
No |
The context used in the authentication. This must be a UTF-8 String no larger than 300 bytes (length may vary dependent on amount of special characters) |
Example request body
{
"device_id" : "64e9e580-7bbe-475d-b99f-3ef52d7012d9",
"context" : "Transaction amount: €2000.-",
"authentication_method" : "OFFLINE_PIN",
"session_expiry_time" : 300000
}
Returns
Returns an offline authentication object if authentication process is successfully initiated. Returns an error object if something goes wrong.
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/offline-authentications' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X POST \
-H 'Content-Type: application/json' \
-d '{
"device_id" : "64e9e580-7bbe-475d-b99f-3ef52d7012d9",
"context" : "Transaction amount: €2000.-",
"authentication_method" : "OFFLINE_PIN",
"session_expiry_time" : 300000
}'
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 911bbfbe-82ad-47e4-bc68-75d671a7b58a
Content-Type: application/json
Content-Length: 591
{
"id" : "bdbe860e-4697-4000-8b2f-84475467f259",
"session_created_time" : "2025-03-17T16:58:08.293UTC",
"session_expiry_time" : "2025-03-17T17:03:08.293UTC",
"session_status" : {
"status" : "IN_PROGRESS",
"description" : "Operation is in progress",
"state" : "IN_PROGRESS"
},
"device_id" : "45fe5f2e-f39f-489f-8540-cb35ef9949e0",
"authentication_method" : "OFFLINE_PIN",
"authentication_level" : "TWO_FACTOR",
"context" : "Transaction amount: €2000.-",
"verification_data" : "1,ze/+Ue+scIsO8Q6uri695A,12,VHJhbnNhY3Rpb24gYW1vdW50OiDigqwyMDAwLi0,6,f751eb63"
}
3.5.3. Verify offline authentication
POST /api/smart-device/v1/offline-authentications/{id}/verifications
To verify an offline authentication session, you send in a one-time-password
Arguments
Path | Type | Required | Description |
---|---|---|---|
|
|
YES |
Client generated OTP to verify |
Example request body
{
"otp" : "123456"
}
Returns
Returns the verification status, and the number of remaining attempts if the call is successful. The status can be offline verification status. Returns an error object if something goes wrong.
Response fields
Field | Type | Description |
---|---|---|
|
|
The result of the authentication. See offline verification status for possible values. |
|
|
Number of attempts before the authentication method gets locked |
Verification status values
Status | Description |
---|---|
|
Operation has completed successfully |
|
Operation failed because the session was cancelled by the device |
|
Operation failed because the session has expired |
|
Operation failed because the device is locked |
|
Operation failed because the authentication method is locked |
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/offline-authentications/106df5cb-22c8-437b-bbe4-f2696db2c583/verifications' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X POST \
-H 'Content-Type: application/json' \
-d '{
"otp" : "123456"
}'
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 4bfc4ad2-2793-49b5-8a1d-20481a4add1b
Content-Type: application/json
Content-Length: 54
{
"status" : "FAILURE",
"remaining_attempts" : 2
}
3.5.4. Get authentication details
GET /api/smart-device/v1/offline-authentications/{id}
Getting the authentication session details lets you know the state of the authentication and all other attributes created as part of the offline-authentication process.
Returns
Returns an offline authentication object if a valid identifier was provided, and returns an error otherwise.
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/offline-authentications/b490d4c9-f712-4689-aa74-29f8c95a49b2' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X GET
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 21d8fd4e-3829-4c2c-a89a-609cfd6bdb60
Content-Type: application/json
Content-Length: 591
{
"id" : "1a68afd0-8b40-42be-91d9-29b48d16eade",
"session_created_time" : "2025-03-17T16:58:08.777UTC",
"session_expiry_time" : "2025-03-17T17:03:08.777UTC",
"session_status" : {
"status" : "IN_PROGRESS",
"description" : "Operation is in progress",
"state" : "IN_PROGRESS"
},
"device_id" : "84a29b09-4ab7-4c58-bd3d-a39d90809e18",
"authentication_method" : "OFFLINE_PIN",
"authentication_level" : "TWO_FACTOR",
"context" : "Transaction amount: €2000.-",
"verification_data" : "1,3HLX5ZZs7f1YVcLpexI2fA,12,VHJhbnNhY3Rpb24gYW1vdW50OiDigqwyMDAwLi0,6,45e88b5d"
}
3.5.5. Cancel authentication
DELETE /api/smart-device/v1/offline-authentications/{id}
To cancel an authentication session in progress thereby preventing the device from finishing the authentication process
Returns
Returns an empty response if the operation succeeds. Returns an error object if something goes wrong.
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/offline-authentications/3e5995b1-b647-4b32-a853-e8e8222ec646' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X DELETE
Example response
HTTP/1.1 204 No Content
X-Correlation-UUID: 3e95a414-5ab5-4753-8f11-7ec6a8c6615d
3.6. Device
Each successful enrollment process registers a new device, this API can retrieve details for a device and deactivate it.
3.6.1. The device object
Field | Type | Description |
---|---|---|
|
|
The UUID for the device |
|
|
The application ID for the device |
|
|
The client API version used in the device’s last operation |
|
|
The client API version that the device was originally activated on |
|
|
Encap server release version that the device was originally activated on |
|
|
SHA256 hash of the unique hardware device ID of the client device. |
|
|
SHA256 hash of the device client salt. |
|
|
The operating system type of the device |
|
|
The device manufacturer |
|
|
The device model |
|
|
List of activated authentication methods (Variant 2). See authentication methods for possible values |
|
|
The time for when the device was originally activated |
|
|
The time for when the device last made an operation |
|
|
An lock object containing lock details. Only present when the device is locked |
|
|
The reference url for the lock |
|
|
List containing all reasons for the device lock. See lock reason for possible values. |
|
|
The push address for the device, this address is used when sending push to the device using FCM or APNS. |
|
|
List of all recoveries for the device. See recovery object for details. |
|
|
Explicit setting for the device to lock/unlock recovery. |
|
|
Attestation object that contains details for all the attestations performed for the device. See attestation object for details. |
3.6.2. Get device details
GET /api/smart-device/v1/devices/{deviceId}
Get device details
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/devices/92c478fc-82d1-48b2-8f91-75c830995d74' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X GET
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 5b95aeec-09ab-4be1-808c-9a16feb4419f
Content-Type: application/json
Content-Length: 1496
{
"id" : "92c478fc-82d1-48b2-8f91-75c830995d74",
"application_id" : "encap",
"current_client_api_version" : "3.20.0",
"activated_client_api_version" : "3.20.0",
"activated_encap_release" : "R_3_20",
"device_hash" : "Xsx6d7bmhh9ovUvhqp5KLOs480%2FWCq3bq9%2FC0apC4TU%3D",
"salt_hash" : "ap7cmrQQTc0zb5aCPgXV0JxvrFeOO6CguQklentLMsc%3D",
"push_address" : "ap7cmrQQTc0zb5aCPgXV0JxvrFeOO6CguQklentLMsc",
"operating_system_type" : "iOS",
"device_manufacturer" : "Apple",
"device_model" : "iPhone14,2",
"activated_authentication_methods" : [ "DEVICE", "DEVICE:PIN" ],
"activation_time" : "2017-04-05T12:23:00.000UTC",
"last_used_time" : "2025-03-17T16:58:10.578UTC",
"lock" : {
"ref" : "/devices/a06563f4-f43e-4cb9-b53a-fe9f77d9fa72/lock",
"lock_reason" : [ "DEVICE_VERIFICATION_FAILED", "LOCKED_BY_ADMIN" ]
},
"attestation" : {
"safety_net_status" : "VERIFIED",
"safety_net_date" : "2025-03-17T16:58:10.577UTC",
"app_attest_status" : "VERIFIED",
"app_attest_date" : "2025-03-17T16:58:10.577UTC",
"play_integrity_status" : "VERIFIED",
"play_integrity_date" : "2025-03-17T16:58:10.577UTC"
},
"recoveries" : [ {
"recovery_id" : "90f3dd41-26a7-40b1-bb60-083690508b95",
"recovery_method" : "CLOUD_BACKUP",
"recovery_status" : "ACTIVATED",
"created_date" : "2025-03-17T16:58:10.578UTC",
"updated_date" : "2025-03-17T16:58:10.578UTC",
"recovered_date" : "2025-03-17T16:58:10.578UTC"
} ],
"recovery_lock" : false
}
3.6.3. Deactivate device
DELETE /api/smart-device/v1/devices/{deviceId}
Disable authentication credentials for this device. This includes deleting device credentials.
Returns
Returns 204 No Content if a valid identifier was provided and the device was deleted. Returns an error object if something goes wrong.
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/devices/92c478fc-82d1-48b2-8f91-75c830995d74' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X DELETE
Example response
HTTP/1.1 204 No Content
X-Correlation-UUID: a636b5a3-16c9-4ec7-8507-c96f9b77b61d
3.7. Device Sessions
All operations for a given enrollment creates a session. A list of all sessions for a device can be retrieved including some meta info for each session. For a more detailed description of a session follow the detailed link in the meta info for that session. For sessions with an operation type without a REST API, the detail link is omitted.
3.7.1. The session object
Field | Type | Description |
---|---|---|
|
|
The UUID for the session |
|
|
Link to authentication object for the session |
|
|
The purpose of the session. See session purpose for details. |
|
|
The state of the session |
|
|
The date for when the session was created. Returned in pattern yyyy-MM-dd’T’HH:mm:ss.SSS’UTC' |
|
|
The date for when the session expires. Returned in pattern yyyy-MM-dd’T’HH:mm:ss.SSS’UTC' |
|
|
True if this is an offline authentication session |
|
|
True if the session was run in client only mode |
|
|
The device ID that owns the session |
3.7.2. Get sessions for device
GET /api/smart-device/v1/device/{deviceId}/sessions?limit=10&offset=0
Returns
Returns a pagination object with a list of session objects if a valid identifier was provided, and returns an error otherwise.
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/devices/92c478fc-82d1-48b2-8f91-75c830995d74/sessions?limit=5' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X GET
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: c0f5eb11-1f3d-4d5d-9ad6-bb853c4abc08
Content-Type: application/json
Content-Length: 1488
{
"previous" : "/smart-device/v1/devices/92c478fc-82d1-48b2-8f91-75c830995d74/sessions?limit=5&offset=dff3245b-6385-47cb-90dd-37166f3ab38c",
"next" : "/smart-device/v1/devices/92c478fc-82d1-48b2-8f91-75c830995d74/sessions?limit=5&offset=e8839236-31bd-461d-af20-f05fe68be14d",
"limit" : 5,
"items" : [ {
"session_id" : "9e668346-6951-4701-bd1f-11a194915472",
"device_id" : "bddcde82-c9be-41e4-977b-da32e5b67dc2",
"ref" : "/enrollments/9e668346-6951-4701-bd1f-11a194915472",
"purpose" : "ACTIVATION",
"state" : "SUCCESS",
"client_only" : false,
"session_created_on" : "2025-03-17T16:58:08.286UTC",
"session_expires_on" : "2025-03-17T16:58:08.286UTC",
"offline" : false
}, {
"session_id" : "b1511bc6-f039-4c47-85ed-40eaf873db59",
"device_id" : "bddcde82-c9be-41e4-977b-da32e5b67dc2",
"ref" : "/authentications/b1511bc6-f039-4c47-85ed-40eaf873db59",
"purpose" : "AUTHENTICATION",
"state" : "IN_PROGRESS",
"client_only" : false,
"session_created_on" : "2025-03-17T16:58:08.286UTC",
"session_expires_on" : "2025-03-17T16:58:08.286UTC",
"offline" : false
}, {
"session_id" : "b1c3f812-36c0-48f6-b083-68ef0c74b512",
"device_id" : "bddcde82-c9be-41e4-977b-da32e5b67dc2",
"purpose" : "DELETE_RECOVERY",
"state" : "IN_PROGRESS",
"client_only" : false,
"session_created_on" : "2025-03-17T16:58:08.286UTC",
"session_expires_on" : "2025-03-17T16:58:08.286UTC",
"offline" : false
} ]
}
3.8. Sessions for multiple devices
Retrieve a list of the latest sessions for a set of devices, including limited meta info for each session. Follow the reference link in the meta info for more detailed description of the session and its meta info. For sessions with an operation type without a REST API, the reference link is omitted. The number of devices to query is limited to 100.
3.8.1. Get sessions for multiple devices
GET /api/smart-device/v1/sessions/sessions?deviceId={deviceId1}&deviceId={deviceId2}&limit=10&offset=0
Returns
Returns a pagination object with a list of session objects if all identifiers are valid, and returns an error otherwise.
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/sessions?deviceId=17ca0b34-f871-4006-9c65-0e9380ce529b&deviceId=f834a828-ee69-4039-adfc-d5b23f33be92&limit=4' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X GET
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: d0cec9cb-9eb9-415e-be1c-6075bb09a6d0
Content-Type: application/json
Content-Length: 2057
{
"previous" : "/smart-device/v1/sessions?deviceId=17ca0b34-f871-4006-9c65-0e9380ce529b&deviceId=f834a828-ee69-4039-adfc-d5b23f33be92&limit=4&offset=49ade620-270e-452a-9bf6-f5c3f8bf8865",
"next" : "/smart-device/v1/sessions?deviceId=17ca0b34-f871-4006-9c65-0e9380ce529b&deviceId=f834a828-ee69-4039-adfc-d5b23f33be92&limit=4&offset=38fad1d3-19d8-481c-b6e5-9a3cda2e6c0f",
"limit" : 4,
"items" : [ {
"session_id" : "69344bbb-bf22-4f1e-9a68-47b573047f22",
"device_id" : "c82e4fea-4c32-4110-ba7e-fd94dcec7da3",
"ref" : "/enrollments/69344bbb-bf22-4f1e-9a68-47b573047f22",
"purpose" : "ACTIVATION",
"state" : "SUCCESS",
"client_only" : false,
"session_created_on" : "2025-03-17T16:58:05.528UTC",
"session_expires_on" : "2025-03-17T16:58:05.528UTC",
"offline" : false
}, {
"session_id" : "f8f96132-6029-46da-aaad-e98dbdfb46a9",
"device_id" : "c82e4fea-4c32-4110-ba7e-fd94dcec7da3",
"ref" : "/authentications/f8f96132-6029-46da-aaad-e98dbdfb46a9",
"purpose" : "AUTHENTICATION",
"state" : "IN_PROGRESS",
"client_only" : false,
"session_created_on" : "2025-03-17T16:58:05.528UTC",
"session_expires_on" : "2025-03-17T16:58:05.528UTC",
"offline" : false
}, {
"session_id" : "90010c7d-a64d-4c14-99fd-a1e23295dc30",
"device_id" : "9a4ea3cb-2870-4d91-8a65-c9b1441a2ba5",
"ref" : "/enrollments/90010c7d-a64d-4c14-99fd-a1e23295dc30",
"purpose" : "ACTIVATION",
"state" : "SUCCESS",
"client_only" : false,
"session_created_on" : "2025-03-17T16:58:05.539UTC",
"session_expires_on" : "2025-03-17T16:58:05.539UTC",
"offline" : false
}, {
"session_id" : "17256cd5-9836-47a6-b85b-eb4d621ed4fc",
"device_id" : "9a4ea3cb-2870-4d91-8a65-c9b1441a2ba5",
"ref" : "/authentications/17256cd5-9836-47a6-b85b-eb4d621ed4fc",
"purpose" : "AUTHENTICATION",
"state" : "IN_PROGRESS",
"client_only" : false,
"session_created_on" : "2025-03-17T16:58:05.539UTC",
"session_expires_on" : "2025-03-17T16:58:05.539UTC",
"offline" : false
} ]
}
3.9. Device Lock
Every device can have a lock. A lock can be created through this API or as a result of various other processes.
3.9.1. The lock object
Field | Type | Description |
---|---|---|
|
|
The UUID for the device |
|
|
An lock object containing lock details |
|
|
The reference url for the lock |
|
|
List containing all reasons for the device lock. See lock reason for possible values. |
3.9.2. Lock device
POST /api/smart-device/v1/devices/{deviceId}/lock
A device is locked by creating a lock on the device-id.
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/devices/92c478fc-82d1-48b2-8f91-75c830995d74/lock' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X POST
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 8c13c538-dbca-407b-9e81-cbad1db94e90
Content-Type: application/json
Content-Length: 207
{
"id" : "92c478fc-82d1-48b2-8f91-75c830995d74",
"lock" : {
"ref" : "/devices/92c478fc-82d1-48b2-8f91-75c830995d74/lock",
"lock_reason" : [ "DEVICE_VERIFICATION_FAILED", "LOCKED_BY_ADMIN" ]
}
}
3.9.3. Get lock status for device
GET /api/smart-device/v1/devices/{deviceId}/lock
Looking at the lock for a device-id will tell why the device was locked.
Returns
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/devices/92c478fc-82d1-48b2-8f91-75c830995d74/lock' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X GET
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 11f1aeb4-0f7a-4509-84db-62d263ddd391
Content-Type: application/json
Content-Length: 207
{
"id" : "92c478fc-82d1-48b2-8f91-75c830995d74",
"lock" : {
"ref" : "/devices/92c478fc-82d1-48b2-8f91-75c830995d74/lock",
"lock_reason" : [ "DEVICE_VERIFICATION_FAILED", "LOCKED_BY_ADMIN" ]
}
}
3.9.4. Unlock device
DELETE /api/smart-device/v1/devices/{deviceId}/lock
A device is unlocked by removing the lock from the device-id.
Returns
Returns 204 No Content if a valid identifier was provided and lock was removed. Returns an error object if something goes wrong.
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/devices/92c478fc-82d1-48b2-8f91-75c830995d74/lock' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X DELETE
Example response
HTTP/1.1 204 No Content
X-Correlation-UUID: e143bb29-db0e-4a01-9f0b-220a19354614
3.10. Lock authentication method
In addition to devices, every authentication method can have a lock. A locked offline-method does not result in the entire device being locked. While locking an online-method will lock the device and make it unable to perform any operation.
See authentication methods for possible values for authMethod (Variant 1).
3.10.1. Lock authentication method
POST /api/smart-device/v1/devices/{deviceId}/authmethods/{authMethod}/lock
Locks the specified authentication method for the device.
Returns
Returns an empty response if the operation succeeds. Returns an error object if something goes wrong.
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/devices/2a7024e0-b5c6-4a42-b7de-67429f837cba/authmethods/OFFLINE_PIN/lock' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X POST
Example response
HTTP/1.1 204 No Content
X-Correlation-UUID: 40e60d9b-8ca0-43a8-9aaf-8f59c97c819b
3.10.2. Get the lock status for the authentication method of the device.
GET /api/smart-device/v1/devices/{deviceId}/authmethods/{authMethod}/lock
Fetches the lock status of the specified authentication method.
Returns
Returns the lock status of the authentication method.
Field | Type | Description |
---|---|---|
|
|
Lock status of this auth method |
Returns an error object if something goes wrong.
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/devices/ed69762a-26dc-474d-87fd-b3907aa87298/authmethods/OFFLINE_PIN/lock' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X GET
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 549234a8-3b18-4fca-aa89-ec3339c4fbee
Content-Type: application/json
Content-Length: 39
{
"lock_status" : "LOCKED_BY_ADMIN"
}
3.10.3. Get the lock status for all authentication methods of the device.
GET /api/smart-device/v1/devices/{deviceId}/authmethods
Gets the lock status for each authentication method that the device has.
Returns
Returns all authentication methods with lock status.
{
"authentication_methods" : [ {
"auth_method" : "DEVICE",
"lock_status" : "OPEN"
}, {
"auth_method" : "DEVICE_PIN",
"lock_status" : "PIN_VERIFICATION_FAILED"
}, {
"auth_method" : "DEVICE_ANDROID_BIOMETRIC_PROMPT",
"lock_status" : "OPEN"
} ]
}
Returns an error object if something goes wrong.
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/devices/9cc91913-5dc1-434e-9430-a36b072aaf68/authmethods' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X GET
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: d2e089b8-1236-4738-8886-a68375a612e0
Content-Type: application/json
Content-Length: 275
{
"authentication_methods" : [ {
"auth_method" : "DEVICE",
"lock_status" : "OPEN"
}, {
"auth_method" : "DEVICE_PIN",
"lock_status" : "PIN_VERIFICATION_FAILED"
}, {
"auth_method" : "DEVICE_ANDROID_BIOMETRIC_PROMPT",
"lock_status" : "OPEN"
} ]
}
3.10.4. Unlock authentication method
DELETE /api/smart-device/v1/devices/{deviceId}/authmethods/{authMethod}/lock
Removes the lock from the authentication method.
Returns
Returns 204 No Content if successful. Returns an error object if something goes wrong.
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/devices/74b0dbbf-760b-45f8-9ce7-11c6801f34ec/authmethods/OFFLINE_PIN/lock' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X DELETE
Example response
HTTP/1.1 204 No Content
X-Correlation-UUID: 9dc3571b-3e7c-49f7-bce7-c8131f1c3478
3.11. Account Recovery
Account Recovery adds support for recovering a device after it being replaced, lost or stolen. With Account Recovery the user can add recovery for his device that is protected with a user created recovery code (PIN). The recovery secret is stored in the cloud backup (Google Cloud or Apple iCloud) provided that the user has enabled backup of his apps. The feature allows the user to recover the app on another device while the service provider reference (device_id) remains unchanged.
3.11.1. The perform recovery session object
Field | Type | Description |
---|---|---|
|
|
The UUID for the recovery session |
|
|
The application ID for the device |
|
|
The UUID for the device |
|
|
The time for when the session was created. Returned in pattern yyyy-MM-dd’T’HH:mm:ss.SSS’UTC' |
|
|
The time for when the session will expire. Returned in pattern yyyy-MM-dd’T’HH:mm:ss.SSS’UTC' |
|
|
The purpose of the session. See session purpose for details. |
|
|
List of activated authentication methods (Variant 2). See authentication methods for possible values |
|
|
The recovery method used to set up the recovery. |
|
|
The recovery status of a given recovery. |
|
|
List that contains name and value for all risk attributes. See risk_attributes object for details. |
|
|
Location object that contains location details, see location object for details. |
|
|
Status object that contains status details of the session. See session_status object for details. |
|
|
Attestation object that contains details for all the attestations performed for the device. See attestation object for details. |
3.11.2. Get perform recovery details
GET /api/smart-device/v1/perform-recoveries/{session_id}
Get the session details after performing a recovery. The session details let you know the state of the recovery, and if finished it gives you access to risk data and all other attributes created as part of the recovery process. You can find the session_id by looking at the device sessions with the correct session purpose.
Returns
Returns an perform recovery object if a valid session identifier was provided, otherwise returns an error.
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/perform-recoveries/9d9ff693-8bc9-485c-a2cb-7b1b531f7151' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X GET
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 0396bbc4-8b8b-4147-91b7-19108441cdb8
Content-Type: application/json
Content-Length: 1435
{
"id" : "9d9ff693-8bc9-485c-a2cb-7b1b531f7151",
"application_id" : "encap",
"device_id" : "4eef37cd-1cce-49a2-a0db-dfbe207877b5",
"session_created_time" : "2025-03-17T16:58:06.745UTC",
"session_expiry_time" : "2025-03-17T18:58:06.745UTC",
"activated_authentication_methods" : [ "DEVICE", "DEVICE:PIN" ],
"location" : {
"accuracy" : "0.564",
"altitude" : "76.2352.3563",
"latitude" : "12.34234.143",
"longitude" : "32.24314.1431"
},
"risk_attributes" : [ {
"name" : "is_debuggable",
"value" : "true"
}, {
"name" : "is_debugger_connected",
"value" : "true"
}, {
"name" : "input_method",
"value" : "input"
}, {
"name" : "is_emulator",
"value" : "true"
}, {
"name" : "operating_system_version",
"value" : "2.3"
}, {
"name" : "client_side_ip",
"value" : "[\"192.168.0.1\"]"
} ],
"session_status" : {
"status" : "IN_PROGRESS",
"description" : "Operation is in progress",
"state" : "IN_PROGRESS"
},
"attestation" : {
"safety_net_status" : "VERIFIED",
"safety_net_date" : "2025-03-17T16:58:06.742UTC",
"app_attest_status" : "VERIFIED",
"app_attest_date" : "2025-03-17T16:58:06.742UTC",
"play_integrity_status" : "VERIFIED",
"play_integrity_date" : "2025-03-17T16:58:06.742UTC"
},
"purpose" : "PERFORM_RECOVERY",
"recovery_method" : "CLOUD_BACKUP_SERVER_SIDE_FACE",
"recovery_status" : "ACTIVATED"
}
3.12. Recovery Lock for device
The Account Recovery feature is enabled in the application configuration and can by default be used by all devices. The feature can be disabled for an explicit device by setting the recovery lock using this API, or by setting the recovery lock field when doing a new enrollment.
The recovery lock is by default set to false and if set to true the device will not be able to perform any recovery operations.
3.12.1. The recovery lock object
Field | Type | Description |
---|---|---|
|
|
Explicit setting for the device to lock/unlock recovery. |
3.12.2. Get recovery lock status for device
GET /api/smart-device/v1/devices/{deviceId}/recovery-lock
Check if recovery is locked for the given device
Returns
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/devices/92c478fc-82d1-48b2-8f91-75c830995d74/recovery-lock' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X GET
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: dc0c0f3e-f9bb-4bdc-94db-ef0f5a16d534
Content-Type: application/json
Content-Length: 29
{
"recovery_lock" : false
}
3.12.3. Change recovery lock
PUT /api/smart-device/v1/devices/{deviceId}/recovery-lock
Lock/unlock recovery for the given device
Returns
Returns 202 Accepted if setting the recovery lock is successful. Returns an error object if something goes wrong.
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/devices/92c478fc-82d1-48b2-8f91-75c830995d74/recovery-lock' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X PUT \
-H 'Content-Type: application/json' \
-d '{
"recovery_lock" : "true"
}'
Example response
HTTP/1.1 202 Accepted
X-Correlation-UUID: 28a9ad6c-aef7-4d51-977e-d5c179952376
Content-Type: application/json
Content-Length: 28
{
"recovery_lock" : true
}
3.13. Override Geofencing settings for device
The Geofencing feature is configured in the application configuration and by default the settings therein applies to all devices. The geofencing configuration for authentication operations can be overridden for an explicit device using this API. By overriding the configuration, the device will have a fully independent configuration (for authentication operations), and the settings in application configuration will no longer apply.
3.13.1. The geofencing object
Field | Type | Description |
---|---|---|
|
|
Geofencing enforcement mode (OFF/OPTIONAL/REQUIRED) |
|
|
Comma separated list of continents in which authentication is allowed (AF/NA/OC/AN/AS/EU/SA) |
|
|
Comma separated list of countries in which authentication is allowed (in addition to allowed continents). ISO 3166-1 alpha-2 standard. |
|
|
Comma separated list of countries in which authentication is not allowed (exception from allowed continents). ISO 3166-1 alpha-2 standard. |
3.13.2. Get geofencing configuration for device
GET /api/smart-device/v1/devices/{deviceId}/geofencing
Retrieve geofencing configuration for the given device
Returns
Returns a geofencing object if a valid device identifier is provided. Returns an error otherwise.
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/devices/92c478fc-82d1-48b2-8f91-75c830995d74/geofencing' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X GET
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 7ae73ea8-f4a0-4f62-b53f-219115ef8a02
Content-Type: application/json
Content-Length: 115
{
"mode" : "OPTIONAL",
"allowed_continents" : "EU",
"allowed_countries" : "CT",
"denied_countries" : "RU"
}
3.13.3. Update geofencing configuration for device
PUT /api/smart-device/v1/devices/{deviceId}/geofencing
Update geofencing configuration for the given device
Returns
Returns 202 Accepted together with the updated geofencing object if the update is successful. Returns an error object if something goes wrong.
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/devices/92c478fc-82d1-48b2-8f91-75c830995d74/geofencing' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X PUT \
-H 'Content-Type: application/json' \
-d '{
"mode" : "OPTIONAL",
"allowed_continents" : "EU",
"allowed_countries" : "CT",
"denied_countries" : "RU"
}'
Example response
HTTP/1.1 202 Accepted
X-Correlation-UUID: 2fe7135a-fc2b-4ddb-9f8d-6b0960e42cc4
Content-Type: application/json
Content-Length: 115
{
"mode" : "OPTIONAL",
"allowed_continents" : "EU",
"allowed_countries" : "CT",
"denied_countries" : "RU"
}
3.13.4. Delete geofencing configuration for device
DELETE /api/smart-device/v1/devices/{deviceId}/geofencing
Delete geofencing configuration for the given device
Returns
Returns 204 No Content if successful. Returns an error object if something goes wrong.
Example request
$ curl 'http://localhost:8080/api/smart-device/v1/devices/92c478fc-82d1-48b2-8f91-75c830995d74/geofencing' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X DELETE
Example response
HTTP/1.1 204 No Content
X-Correlation-UUID: 4feddb4b-2e8b-47a2-88cf-62a5c465d96d
4. Admin API
4.1. Server status
Provides a health check of the Encap instances. If called unauthenticated only a overall status is provided.
4.1.1. The status object
Field | Type | Description |
---|---|---|
|
|
Indicates if the API is in a "healthy" state |
|
|
An RFC 3339 formatted date and time in UTC |
|
|
Array of underlying dependencies and their status. Only included if request is authenticated |
|
|
Indicates if this dependency is in a "healthy" state |
|
|
Time in milliseconds it took to check this dependency |
|
|
Name of dependency |
4.1.2. Get API status
GET /api/admin/v1/status
Example request
$ curl 'http://localhost:8080/api/admin/v1/status' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X GET
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 815df510-853b-4e6f-8dd5-153ec19d6740
Content-Type: application/json
Content-Length: 179
{
"success" : true,
"created_on" : "2025-03-17T16:58:03.188UTC",
"dependencies" : [ {
"resource" : "Core database",
"success" : true,
"request_time" : 10
} ]
}
Example response if unauthenticated
HTTP/1.1 200 OK
X-Correlation-UUID: 24fcc7dc-0bd0-4468-991e-5892a36394f0
Content-Type: application/json
Content-Length: 93
{
"success" : true,
"created_on" : "2025-03-17T16:58:03.775UTC",
"dependencies" : [ ]
}
4.2. Application Configuration
Operations on Application Configuration.
Consult 3.1.2 - ApplicationID configurations in the Encap Server Administration Manual for information about this topic.
All operations on this endpoint requires authentication. See the rest api authentication section
4.2.1. The application configuration object
Field | Type | Description |
---|---|---|
|
|
The logical name of the application configuration |
|
|
The unique ID of this application configuration registration. This value is central to these APIs |
|
|
Reference to the APNS push certificate or token. |
|
|
The human readable name for the application configuration |
|
|
The application configuration parameters |
4.2.2. The configuration object
This object contains the configuration parameters and their value
Parameter | Description | Allowed values | Default |
---|---|---|---|
|
Lower limit for how guessable the activation code is. Given as 1 in x where this param represents x. The server calculates the actual value based on the cardinality of the activation code type, the activation code length and the number of active activations. If this value is below 1 in x with x as defined here the server prevents the new activation from starting. It is not possible to set this lower than 1 in 1,000. The higher the number the lower the probability is of guessing the activation code. To increase the number of concurrently active activation supported increase the ACTIVATION_CODE_LENGTH or choose a ACTIVATION_CODE_TYPE with greater cardinality. E.g. ACTIVATION_CODE_LENGTH = 4 ACTIVATION_CODE_TYPE = NUMERIC This gives 10,000 possible activation codes. With 1 activation in progress the chance of guessing the activation code is 1 in 10,000. With 10 concurrent activations we have a chance of guessing a valid code reduced to 1 in 1,000 (10,000 / 10 = 1,000). If an 11th activation session starts before any of the others finish it will fail the ACTIVATION_CODE_ALLOWED_GUESS_PROBABILITY check as this brings the chance below 1 in 1,000 (10,000 / 11 = 909). |
1000 .. MAXINT |
1000 |
|
The length in characters of the activation code that should be generated. |
4 .. MAXINT |
6 |
|
The type of characters that can be used during the generation of the activation code. |
ALPHA, ALPHANUMERIC, ANY, NUMERIC |
NUMERIC |
|
Comma separated list of allowed authentication methods. Determines which auth methods can be activated and used for authentication |
See authentication methods for applicable auth methods (Variant 1) |
DEVICE, DEVICE:PIN |
|
Comma separated list of allowed authentication methods for activation of a new auth-method. Determines which auth methods can be used to authenticate during activation of a new auth method. The value(s) here must be present in the ALLOWED_AUTH_METHODS parameter. Offline authentication methods can not be used here. |
See authentication methods for applicable auth methods (Variant 1) |
DEVICE:PIN |
|
The grace amount of failed DEVICE_PIN (or offline) authentications for any client before they are locked out. |
0..MAXINT |
3 |
|
The APN server configuration that defines where to reach the APNs. |
PRODUCTION, SANDBOX |
PRODUCTION |
|
The expiration time for an APNs message, set in milliseconds, indicates how long APNs should try to deliver the message. If not delivered by this time, the message is discarded, although APNs attempts delivery at least once regardless of the expiration time. |
0..MAXINT |
86400000 |
|
Apples bundle id for the application. Used as topic on the push message sent to APNs, required when using APNs provider token for sending push messages. |
String |
|
|
Enable notification sound for push messages to iOS devices. |
true, false |
false |
|
Sets the interruption level for push messages to iOS devices to "time-sensitive". |
true, false |
false |
|
True if the client is allowed to perform client-only activations and authentications. A client-only operation is one that is not initiated by a service provider request. |
true, false |
true |
|
Android SDK API versions to blacklist (and reject). See the android configuration chapter in the server manual for smart device for details. |
Comma separated semantic version, ex: "3.5.3, 3.6.8" |
null |
|
IOS SDK API versions to blacklist (and reject). See the ios configuration chapter in the server manual for smart device for details. |
Comma separated semantic version, ex: "3.5.3, 3.6.8" |
null |
|
Comma separated list of which risk data to store, or ALL if all the available risk data should be stored. Details for risk data is specified in the EncapAuthenticationStateless document and in the REST documentation. |
See config attribute column in risk_attributes for available values. Value 'ALL' will enable all risk attributes. |
Default value is empty which represents that no risk data is enabled. |
|
Firebase Cloud Messaging. The contents of the serviceAccount.json (credentials file) for your Firebase Cloud Messaging project. |
String |
|
|
Firebase Cloud Messaging. Maximum lifespan of the message in milliseconds. The default value is 0. This means deliver “now or never”. FCM guarantees best effort for messages with this lifespan. Maximum duration is 4 weeks. |
0..MAXINT |
0 |
|
What to do if validating the signature based on the hardware supported keys. SUPPORTED means that clients who register the public key in a healthy manner during Registration, will fail Authentication if they fail to provide a signature based on the Hardware Supported key. If this flag is RISK_PARAMS, the failing signature will only result in risk parameters on authentication and will not fail the authentication. |
SUPPORTED RISK_PARAMS |
SUPPORTED |
|
The amount of time (in milliseconds) that a new client session remains valid for. After this time has elapsed, the session can no longer be used for any operations |
1..MAXINT |
300000 |
|
The maximum amount of time (in milliseconds) the sessionExpiry can be set to. |
1..MAXINT |
300000 |
|
What Android client SDK version should be allowed. This can be used to narrow (not extend) the SDK version. Example is if the server minimum is "3.15.0", and someone wants to only allow "3.16.0" -clients, this can be achieved here. But putting "3.13.0" will not have any effect. |
Semantic version, ex: "3.17.0" |
null |
|
The same as MINIMUM_REQUIRED_ENCAP_API_VERSION_ANDROID, but applies to iOS clients. |
Semantic version, ex: "3.17.0" |
null |
|
Enable the server to send push messages with Fire Cloud Messaging or Apple APNS |
true, false |
false |
|
Enable users to set up recovery with an alternative set of user credentials |
true, false |
false |
|
The minimum number of characters for the recovery PIN. Note: This is a hint to the client and not enforced by the server. |
0..MAXINT |
6 |
|
The maximum number of characters for the recovery PIN. Note: This is a hint to the client and not enforced by the server. |
0..MAXINT |
50 |
|
The type of characters that can be used in the recovery PIN. Note: This is a hint to the client and not enforced by the server. |
ALPHA, ALPHANUMERIC, ANY, NUMERIC |
NUMERIC |
|
The grace amount of failed recovery code attempts for any client before the recovery for the client is locked. |
0..MAXINT |
3 |
|
Authorization token timeout in milliseconds. After this time, the token will not be valid anymore. |
0..MAXINT |
300000 |
|
Geofencing mode for activation. REQUIRED: Device location check is performed. If the location is not part of the allowed regions or the location check fails, activation request will fail. OPTIONAL: Device location check is performed. If the location is not part of the allowed regions or the location check fails, activation request will be performed and Service Provider will be notified about it via the API. OFF: Device location check is not performed. |
REQUIRED, OPTIONAL, OFF |
OFF |
|
List of continents in which activation is allowed. |
2 letter continent codes (AF/NA/OC/AN/AS/EU/SA), in a comma separated list. |
|
|
List of countries in which activation is allowed in addition to allowed continents. |
Country codes following ISO 3166-1 alpha-2 standard, in a comma separated list. |
|
|
List of countries in which activation is not allowed (exception from allowed continents). |
Country codes following ISO 3166-1 alpha-2 standard, in a comma separated list. |
|
|
Geofencing mode for authentication. REQUIRED: Device location check is performed. If the location is not part of the allowed regions or the location check fails, authentication request will fail and Service Provider will be notified about it via the API. OPTIONAL: Device location check is performed. If the location is not part of the allowed regions or the location check fails, authentication request will be performed. OFF: Device location check is not performed. |
REQUIRED, OPTIONAL, OFF |
OFF |
|
List of continents in which authentication is allowed. |
2 letter continent codes (AF/NA/OC/AN/AS/EU/SA), in a comma separated list. |
|
|
List of countries in which authentication is allowed in addition to allowed continents. |
Country codes following ISO 3166-1 alpha-2 standard, in a comma separated list. |
|
|
List of countries in which authentication is not allowed (exception from allowed continents). |
Country codes following ISO 3166-1 alpha-2 standard, in a comma separated list. |
|
|
Timeout allowed for the client to wait for location and perform reverse geolocation lookup (if the timeout is exceeded, the client will continue without country). |
0..MAXINT |
10000 |
|
Comma separated list of events that shall trigger a REST callback to the service provider url configured. You can also filter events per API to have even more control of when a callback should be issued. Allowed events: See event-callback-events When not specifying an API the callback for the event will be sent regardless of API. e.g. DEVICE_LOCKED, DEVICE_DEACTIVATED:[REST] Format specification: <event-type-1>[:< [api-1|api-2|…] >], <event-type-2>[:< [api-1|api-2|…] >] |
Allowed events: See event-callback-events |
|
|
The URL that should receive callbacks in case any callback events have been configured for this app-config. |
String |
|
|
Comma separated list of operating system types (iOS/Android) for enabling client debug data. It might be set to one of the platforms, or both. It is empty by default, hence no client debug data is sent to the server. |
IOS, ANDROID |
|
|
The minimum length in characters of the PIN. Note: This is a hint to the client and not enforced by the server (but enforced in the client SDK). |
1..MAXINT |
4 |
|
The maximum length in characters of the PIN. Note: This is a hint to the client and not enforced by the server (but enforced in the client SDK). |
1..MAXINT |
4 |
|
The type of characters that can be used in the PIN. Note: This is a hint to the client and not enforced by the server. |
ANY, NUMERIC, ALPHA, ALPHANUMERIC |
NUMERIC |
|
The type of token to respond with to the client when one is requested. Note: The client can request a different type of token during the authentication operation. This setting indicates the default type to use when the client doesn’t specify one. |
SAML1, SAML2 |
SAML2 |
|
The scheme part of URLs generated for push methods that require a URL. This is the URL Scheme that the client is configured to be activated with. |
A valid URL scheme |
encap |
|
SafetyNet Attestation mode. REQUIRED: SafetyNet attestation is preformed. If attestation fails activation/authentication request will fail. OPTIONAL: SafetyNet attestation is preformed. If attestation fails activation/authentication request will not fail and a new attestation is performed on the next request. OFF: SafetyNet attestation is not preformed. The SafetyNet API has been deprecated by Google and is now replaced by the Play Integrity API which is supported from Encap 3.17. |
REQUIRED, OPTIONAL, OFF |
OFF |
|
SafetyNet Attestation integrity mode. Defines the strictness when interpreting the SafetyNet attestation. Required if ATTESTATION_ANDROID_SAFETYNET_MODE is REQUIRED or OPTIONAL. LEAN: Requires basicIntegrity integrity to be true. STRICT: Requires both ctsProfileMatch and basicIntegrity to be true. |
LEAN, STRICT |
|
|
SafetyNet Attestation timeout, in milliseconds, the timeout for a request made to SafetyNet. Required if ATTESTATION_ANDROID_SAFETYNET_MODE is REQUIRED or OPTIONAL. |
1..MAXINT |
|
|
Play Integrity attestation mode. REQUIRED: Play Integrity attestation is preformed. If attestation fails activation/authentication request will fail. OPTIONAL: Play Integrity attestation is preformed. If attestation fails activation/authentication request will not fail and a new attestation is performed on the next request. OFF: Play Integrity attestation is not preformed. Play Integrity attestation was introduced in version 3.17 and will only be applicable for clients 3.17 or newer. |
REQUIRED, OPTIONAL, OFF |
OFF |
|
Play Integrity attestation timeout, in milliseconds, the timeout for a request made to Play Integrity. Required if ATTESTATION_ANDROID_PLAY_INTEGRITY_MODE is REQUIRED or OPTIONAL. |
1..MAXINT |
|
|
Play Integrity attestation decryption key, used to decrypt the integrity token. Required if ATTESTATION_ANDROID_PLAY_INTEGRITY_MODE is REQUIRED or OPTIONAL. |
Base64 |
|
|
Play Integrity attestation verification key, used to validate the integrity token. Required if ATTESTATION_ANDROID_PLAY_INTEGRITY_MODE is REQUIRED or OPTIONAL. |
Base64 |
|
|
Play Integrity/SafetyNet attestation, the APK package name. Required if ATTESTATION_ANDROID_PLAY_INTEGRITY_MODE or ATTESTATION_ANDROID_SAFETYNET_MODE is REQUIRED or OPTIONAL. |
String |
|
|
SafetyNet Attestation, the APK certificate SHA-256 digest. Base64 encoded SHA-256. Required if ATTESTATION_ANDROID_SAFETYNET_MODE is REQUIRED or OPTIONAL. |
Base64 |
|
|
iOS Attestation mode when using Apple App Attest. REQUIRED: App attestation enabled. If attestation fails the activation/authentication request will fail. Note that devices running versions of iOS older than 14 will always fail if the mode is set to REQUIRED, due to requirements by the Apple App Attest API. OPTIONAL: App attestation enabled. Even if the attestation fails, the activation/authentication request will succeed, and a new attestation will be performed on the next request. The status can be seen in the response object. OFF: App attestation disabled. |
REQUIRED, OPTIONAL, OFF |
OFF |
|
The environment for an app that uses the App Attest service to validate itself. |
DEVELOPMENT, PRODUCTION |
PRODUCTION |
|
iOS app attestation timeout, after this time, in milliseconds, the attestation request will time out. |
1..MAXINT |
20000 |
|
App ID which is a concatenation of a 10-digit team identifier, a period, and the app’s CFBundleIdentifier value. Required when using Apple App Attest service. |
String |
|
|
Validation of client request time to be able to block client requests coming in to the server out of order. Recommending to set as enabled, and should only be disabled in case of an error or for debugging purposes. |
true, false |
true |
|
Perform validation of the client request time if the new request is within the threshold time of the last one. Recommended to set equal or higher to the load balancer idle timeout. |
1..MAXINT |
300000 |
|
Length of one time password. Required if any of the OTP authentication methods are available. |
4..9 |
|
|
This setting determines the lock behavior following failed authentication attempts. AUTH_METHOD: Only the current auth method will be locked. This allows the user to unlock it using another valid, unlocked method. DEVICE: The entire device will be locked, rendering all authentication methods unusable. In this case, the service provider must unlock the device using the device admin REST API. |
AUTH_METHOD, DEVICE |
AUTH_METHOD |
|
Server Side Face OAuth2 credentials. The base64 encoded clientId:clientSecret provided when signing up for Server Side Face feature |
Base64 |
4.2.3. Create application configuration
POST /api/admin/v1/appconfig
Creates a new application configuration. Returns the application configuration object.
Arguments
Path | Type | Required | Description |
---|---|---|---|
|
|
Yes |
The logical name of the application configuration |
|
|
No |
The UUID of the APNs push certificate or token used. Required if NATIVE_PUSH_ENABLED is true. |
|
|
No |
The human readable name for the application configuration |
|
|
Yes |
The application configuration parameters. Required to contain at least one configuration. |
Returns
Returns an application configuration object if request is process successfully. Returns an error object if something goes wrong.
Example request
$ curl 'http://localhost:8080/api/admin/v1/appconfig/' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X POST \
-H 'Content-Type: application/json' \
-d '{
"app_id" : "some-new-app",
"apns_uuid" : "f30d8fce-f65c-42be-b6d2-9d271e2192ed",
"app_name" : "AppName",
"configuration" : {
"CLIENT_REQUEST_TIME_VALIDATION_THRESHOLD" : "300000",
"RECOVERY_CODE_MIN_LENGTH" : "6",
"GEOFENCING_ACTIVATION_MODE" : "OFF",
"ATTESTATION_IOS_APP_ATTEST_TIMEOUT" : "20000",
"ATTESTATION_IOS_APP_ATTEST_ENVIRONMENT" : "PRODUCTION",
"RECOVERY_CODE_MAX_LENGTH" : "50",
"ALLOWED_AUTH_METHODS" : "\n DEVICE,\n DEVICE:PIN\n ",
"HWKEY_VALIDATION_STRATEGY" : "SUPPORTED",
"GEOFENCING_AUTHENTICATION_ALLOWED_CONTINENTS" : "",
"MAXIMUM_SESSION_EXPIRY" : "300000",
"SESSION_EXPIRY" : "300000",
"RECOVERY_ENABLED" : "false",
"RECOVERY_CODE_FORMAT" : "NUMERIC",
"MAX_PIN_CODE_LENGTH" : "6",
"TOKEN_TYPE" : "SAML2",
"AUTHORIZATION_TOKEN_TIMEOUT" : "300000",
"GEOFENCING_AUTHENTICATION_ALLOWED_COUNTRIES" : "",
"URL_SCHEME" : "encap",
"CLIENT_ONLY_ALLOWED" : "true",
"GEOFENCING_ACTIVATION_ALLOWED_CONTINENTS" : "",
"ATTESTATION_IOS_APP_ATTEST_MODE" : "OFF",
"ATTESTATION_ANDROID_SAFETYNET_MODE" : "OFF",
"APN_EXPIRY" : "86400000",
"APNS_TIME_SENSITIVE_INTERRUPTION_LEVEL_ENABLED" : "false",
"GEOFENCING_AUTHENTICATION_MODE" : "OFF",
"APN_CONFIG" : "PRODUCTION",
"CLIENT_REQUEST_TIME_VALIDATION_ENABLED" : "true",
"ACTIVATION_CODE_TYPE" : "NUMERIC",
"AMOUNT_FAILURES_ALLOWED" : "3",
"GEOFENCING_AUTHENTICATION_DENIED_COUNTRIES" : "",
"ACTIVATION_CODE_ALLOWED_GUESS_PROBABILITY" : "1000",
"ATTESTATION_ANDROID_PLAY_INTEGRITY_MODE" : "OFF",
"APNS_NOTIFICATION_SOUND_ENABLED" : "false",
"CLIENT_DEBUG_DATA_ENABLED_OS_TYPES" : "",
"GEOFENCING_TIMEOUT" : "10000",
"PIN_CODE_LENGTH" : "4",
"GEOFENCING_ACTIVATION_ALLOWED_COUNTRIES" : "",
"ACTIVATION_CODE_LENGTH" : "6",
"PIN_CODE_TYPE" : "NUMERIC",
"GEOFENCING_ACTIVATION_DENIED_COUNTRIES" : "",
"NATIVE_PUSH_ENABLED" : "true",
"RECOVERY_CODE_AMOUNT_FAILURES_ALLOWED" : "3",
"FIREBASE_TIME_TO_LIVE" : "0",
"ATTESTATION_IOS_APP_ATTEST_APP_ID" : "",
"ALLOWED_AUTH_METHODS_FOR_AUTH_AND_ACTIVATE" : "\n DEVICE:PIN\n ",
"LOCK_SCOPE" : "AUTH_METHOD",
"ENABLED_RISK_DATA" : "ALL"
}
}'
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: d6951736-010b-40a4-b892-092effa8511b
Content-Type: application/json
Content-Length: 2283
{
"app_id" : "some-new-app",
"uuid" : "b7a67fad-590d-4ce7-910c-dec6ef8efea4",
"apns_uuid" : "f30d8fce-f65c-42be-b6d2-9d271e2192ed",
"configuration" : {
"CLIENT_REQUEST_TIME_VALIDATION_THRESHOLD" : "300000",
"RECOVERY_CODE_MIN_LENGTH" : "6",
"GEOFENCING_ACTIVATION_MODE" : "OFF",
"ATTESTATION_IOS_APP_ATTEST_TIMEOUT" : "20000",
"ATTESTATION_IOS_APP_ATTEST_ENVIRONMENT" : "PRODUCTION",
"RECOVERY_CODE_MAX_LENGTH" : "50",
"ALLOWED_AUTH_METHODS" : "\n DEVICE,\n DEVICE:PIN\n ",
"HWKEY_VALIDATION_STRATEGY" : "SUPPORTED",
"GEOFENCING_AUTHENTICATION_ALLOWED_CONTINENTS" : "",
"MAXIMUM_SESSION_EXPIRY" : "300000",
"SESSION_EXPIRY" : "300000",
"RECOVERY_ENABLED" : "false",
"RECOVERY_CODE_FORMAT" : "NUMERIC",
"MAX_PIN_CODE_LENGTH" : "6",
"TOKEN_TYPE" : "SAML2",
"AUTHORIZATION_TOKEN_TIMEOUT" : "300000",
"GEOFENCING_AUTHENTICATION_ALLOWED_COUNTRIES" : "",
"URL_SCHEME" : "encap",
"CLIENT_ONLY_ALLOWED" : "true",
"GEOFENCING_ACTIVATION_ALLOWED_CONTINENTS" : "",
"ATTESTATION_IOS_APP_ATTEST_MODE" : "OFF",
"ATTESTATION_ANDROID_SAFETYNET_MODE" : "OFF",
"APN_EXPIRY" : "86400000",
"APNS_TIME_SENSITIVE_INTERRUPTION_LEVEL_ENABLED" : "false",
"GEOFENCING_AUTHENTICATION_MODE" : "OFF",
"APN_CONFIG" : "PRODUCTION",
"CLIENT_REQUEST_TIME_VALIDATION_ENABLED" : "true",
"ACTIVATION_CODE_TYPE" : "NUMERIC",
"AMOUNT_FAILURES_ALLOWED" : "3",
"GEOFENCING_AUTHENTICATION_DENIED_COUNTRIES" : "",
"ACTIVATION_CODE_ALLOWED_GUESS_PROBABILITY" : "1000",
"ATTESTATION_ANDROID_PLAY_INTEGRITY_MODE" : "OFF",
"APNS_NOTIFICATION_SOUND_ENABLED" : "false",
"CLIENT_DEBUG_DATA_ENABLED_OS_TYPES" : "",
"GEOFENCING_TIMEOUT" : "10000",
"PIN_CODE_LENGTH" : "4",
"GEOFENCING_ACTIVATION_ALLOWED_COUNTRIES" : "",
"ACTIVATION_CODE_LENGTH" : "6",
"PIN_CODE_TYPE" : "NUMERIC",
"GEOFENCING_ACTIVATION_DENIED_COUNTRIES" : "",
"NATIVE_PUSH_ENABLED" : "true",
"RECOVERY_CODE_AMOUNT_FAILURES_ALLOWED" : "3",
"FIREBASE_TIME_TO_LIVE" : "0",
"ATTESTATION_IOS_APP_ATTEST_APP_ID" : "",
"ALLOWED_AUTH_METHODS_FOR_AUTH_AND_ACTIVATE" : "\n DEVICE:PIN\n ",
"LOCK_SCOPE" : "AUTH_METHOD",
"ENABLED_RISK_DATA" : "ALL"
}
}
4.2.4. Get application configuration
GET /api/admin/v1/appconfig/{uuid}
Returns the application configuration object with the uuid.
Example request
$ curl 'http://localhost:8080/api/admin/v1/appconfig/536cfa52-1ba1-4454-b69f-aeeb6ec7d4a1' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X GET \
-H 'Content-Type: application/json'
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: d1e4c0df-6e25-4fe4-a472-085eb41e0f5c
Content-Type: application/json
Content-Length: 2315
{
"app_id" : "encapApiTest",
"uuid" : "536cfa52-1ba1-4454-b69f-aeeb6ec7d4a1",
"apns_uuid" : "c5c60db7-5f1b-4b6a-8dcc-ed8dd5c746fa",
"app_name" : "applicationName",
"configuration" : {
"ACTIVATION_CODE_ALLOWED_GUESS_PROBABILITY" : "1000",
"ACTIVATION_CODE_LENGTH" : "6",
"ACTIVATION_CODE_TYPE" : "NUMERIC",
"ALLOWED_AUTH_METHODS" : "\n DEVICE,\n DEVICE:PIN\n ",
"ALLOWED_AUTH_METHODS_FOR_AUTH_AND_ACTIVATE" : "\n DEVICE:PIN\n ",
"AMOUNT_FAILURES_ALLOWED" : "3",
"APNS_NOTIFICATION_SOUND_ENABLED" : "false",
"APNS_TIME_SENSITIVE_INTERRUPTION_LEVEL_ENABLED" : "false",
"APN_CONFIG" : "PRODUCTION",
"APN_EXPIRY" : "86400000",
"ATTESTATION_ANDROID_PLAY_INTEGRITY_MODE" : "OFF",
"ATTESTATION_ANDROID_SAFETYNET_MODE" : "OFF",
"ATTESTATION_IOS_APP_ATTEST_APP_ID" : "",
"ATTESTATION_IOS_APP_ATTEST_ENVIRONMENT" : "PRODUCTION",
"ATTESTATION_IOS_APP_ATTEST_MODE" : "OFF",
"ATTESTATION_IOS_APP_ATTEST_TIMEOUT" : "20000",
"AUTHORIZATION_TOKEN_TIMEOUT" : "300000",
"CLIENT_DEBUG_DATA_ENABLED_OS_TYPES" : "",
"CLIENT_ONLY_ALLOWED" : "true",
"CLIENT_REQUEST_TIME_VALIDATION_ENABLED" : "true",
"CLIENT_REQUEST_TIME_VALIDATION_THRESHOLD" : "300000",
"ENABLED_RISK_DATA" : "",
"FIREBASE_TIME_TO_LIVE" : "0",
"GEOFENCING_ACTIVATION_ALLOWED_CONTINENTS" : "",
"GEOFENCING_ACTIVATION_ALLOWED_COUNTRIES" : "",
"GEOFENCING_ACTIVATION_DENIED_COUNTRIES" : "",
"GEOFENCING_ACTIVATION_MODE" : "OFF",
"GEOFENCING_AUTHENTICATION_ALLOWED_CONTINENTS" : "",
"GEOFENCING_AUTHENTICATION_ALLOWED_COUNTRIES" : "",
"GEOFENCING_AUTHENTICATION_DENIED_COUNTRIES" : "",
"GEOFENCING_AUTHENTICATION_MODE" : "OFF",
"GEOFENCING_TIMEOUT" : "10000",
"HWKEY_VALIDATION_STRATEGY" : "SUPPORTED",
"LOCK_SCOPE" : "AUTH_METHOD",
"MAXIMUM_SESSION_EXPIRY" : "300000",
"MAX_PIN_CODE_LENGTH" : "4",
"NATIVE_PUSH_ENABLED" : "false",
"PIN_CODE_LENGTH" : "4",
"PIN_CODE_TYPE" : "NUMERIC",
"RECOVERY_CODE_AMOUNT_FAILURES_ALLOWED" : "3",
"RECOVERY_CODE_FORMAT" : "NUMERIC",
"RECOVERY_CODE_MAX_LENGTH" : "50",
"RECOVERY_CODE_MIN_LENGTH" : "6",
"RECOVERY_ENABLED" : "false",
"SESSION_EXPIRY" : "300000",
"TOKEN_TYPE" : "SAML2",
"URL_SCHEME" : "encap"
}
}
4.2.5. Get all application configurations
GET /api/admin/v1/appconfig?limit=10&offset=0
Returns a pagination object with a list of application configuration objects that belongs to your organization.
Example request
$ curl 'http://localhost:8080/api/admin/v1/appconfig?limit=3&offset=63508d58-cc17-46d3-95fa-25ae3ff56f67' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X GET
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 1e896d55-d89e-43a5-87d7-c9c4001180af
Content-Type: application/json
Content-Length: 7627
{
"previous" : "/admin/v1/appconfig?limit=3&offset=89d37e6c-9dea-48b2-b8c6-c52752da81ea",
"next" : "/admin/v1/appconfig?limit=3&offset=bcafd83d-5482-40bf-b855-a2eca31fcbcc",
"offset" : "63508d58-cc17-46d3-95fa-25ae3ff56f67",
"limit" : 3,
"items" : [ {
"app_id" : "Application-0",
"uuid" : "2b72c8ac-9e62-4d96-9db9-926f280e96ac",
"apns_uuid" : "c47a25fc-5e89-4161-9051-270fd20ee172",
"app_name" : "Application Name 0",
"status" : "ENABLED",
"configuration" : {
"CLIENT_REQUEST_TIME_VALIDATION_THRESHOLD" : "300000",
"RECOVERY_CODE_MIN_LENGTH" : "6",
"GEOFENCING_ACTIVATION_MODE" : "OFF",
"ATTESTATION_IOS_APP_ATTEST_TIMEOUT" : "20000",
"ATTESTATION_IOS_APP_ATTEST_ENVIRONMENT" : "PRODUCTION",
"RECOVERY_CODE_MAX_LENGTH" : "50",
"ALLOWED_AUTH_METHODS" : "\n DEVICE,\n DEVICE:PIN\n ",
"HWKEY_VALIDATION_STRATEGY" : "SUPPORTED",
"GEOFENCING_AUTHENTICATION_ALLOWED_CONTINENTS" : "",
"MAXIMUM_SESSION_EXPIRY" : "300000",
"SESSION_EXPIRY" : "300000",
"RECOVERY_ENABLED" : "false",
"RECOVERY_CODE_FORMAT" : "NUMERIC",
"MAX_PIN_CODE_LENGTH" : "4",
"TOKEN_TYPE" : "SAML2",
"AUTHORIZATION_TOKEN_TIMEOUT" : "300000",
"GEOFENCING_AUTHENTICATION_ALLOWED_COUNTRIES" : "",
"URL_SCHEME" : "encap",
"CLIENT_ONLY_ALLOWED" : "true",
"GEOFENCING_ACTIVATION_ALLOWED_CONTINENTS" : "",
"ATTESTATION_IOS_APP_ATTEST_MODE" : "OFF",
"ATTESTATION_ANDROID_SAFETYNET_MODE" : "OFF",
"APN_EXPIRY" : "86400000",
"APNS_TIME_SENSITIVE_INTERRUPTION_LEVEL_ENABLED" : "false",
"GEOFENCING_AUTHENTICATION_MODE" : "OFF",
"APN_CONFIG" : "PRODUCTION",
"CLIENT_REQUEST_TIME_VALIDATION_ENABLED" : "true",
"ACTIVATION_CODE_TYPE" : "NUMERIC",
"AMOUNT_FAILURES_ALLOWED" : "3",
"GEOFENCING_AUTHENTICATION_DENIED_COUNTRIES" : "",
"ACTIVATION_CODE_ALLOWED_GUESS_PROBABILITY" : "1000",
"ATTESTATION_ANDROID_PLAY_INTEGRITY_MODE" : "OFF",
"APNS_NOTIFICATION_SOUND_ENABLED" : "false",
"CLIENT_DEBUG_DATA_ENABLED_OS_TYPES" : "",
"GEOFENCING_TIMEOUT" : "10000",
"PIN_CODE_LENGTH" : "4",
"GEOFENCING_ACTIVATION_ALLOWED_COUNTRIES" : "",
"ACTIVATION_CODE_LENGTH" : "6",
"PIN_CODE_TYPE" : "NUMERIC",
"GEOFENCING_ACTIVATION_DENIED_COUNTRIES" : "",
"NATIVE_PUSH_ENABLED" : "false",
"RECOVERY_CODE_AMOUNT_FAILURES_ALLOWED" : "3",
"FIREBASE_TIME_TO_LIVE" : "0",
"ATTESTATION_IOS_APP_ATTEST_APP_ID" : "",
"ALLOWED_AUTH_METHODS_FOR_AUTH_AND_ACTIVATE" : "\n DEVICE:PIN\n ",
"LOCK_SCOPE" : "AUTH_METHOD",
"ENABLED_RISK_DATA" : ""
}
}, {
"app_id" : "Application-1",
"uuid" : "a81ae6f6-0b24-4bee-96e0-a31473244ce1",
"apns_uuid" : "9bfab921-64ed-49a0-b031-91c4aa295a5d",
"app_name" : "Application Name 1",
"status" : "ENABLED",
"configuration" : {
"CLIENT_REQUEST_TIME_VALIDATION_THRESHOLD" : "300000",
"RECOVERY_CODE_MIN_LENGTH" : "6",
"GEOFENCING_ACTIVATION_MODE" : "OFF",
"ATTESTATION_IOS_APP_ATTEST_TIMEOUT" : "20000",
"ATTESTATION_IOS_APP_ATTEST_ENVIRONMENT" : "PRODUCTION",
"RECOVERY_CODE_MAX_LENGTH" : "50",
"ALLOWED_AUTH_METHODS" : "\n DEVICE,\n DEVICE:PIN\n ",
"HWKEY_VALIDATION_STRATEGY" : "SUPPORTED",
"GEOFENCING_AUTHENTICATION_ALLOWED_CONTINENTS" : "",
"MAXIMUM_SESSION_EXPIRY" : "300000",
"SESSION_EXPIRY" : "300000",
"RECOVERY_ENABLED" : "false",
"RECOVERY_CODE_FORMAT" : "NUMERIC",
"MAX_PIN_CODE_LENGTH" : "4",
"TOKEN_TYPE" : "SAML2",
"AUTHORIZATION_TOKEN_TIMEOUT" : "300000",
"GEOFENCING_AUTHENTICATION_ALLOWED_COUNTRIES" : "",
"URL_SCHEME" : "encap",
"CLIENT_ONLY_ALLOWED" : "true",
"GEOFENCING_ACTIVATION_ALLOWED_CONTINENTS" : "",
"ATTESTATION_IOS_APP_ATTEST_MODE" : "OFF",
"ATTESTATION_ANDROID_SAFETYNET_MODE" : "OFF",
"APN_EXPIRY" : "86400000",
"APNS_TIME_SENSITIVE_INTERRUPTION_LEVEL_ENABLED" : "false",
"GEOFENCING_AUTHENTICATION_MODE" : "OFF",
"APN_CONFIG" : "PRODUCTION",
"CLIENT_REQUEST_TIME_VALIDATION_ENABLED" : "true",
"ACTIVATION_CODE_TYPE" : "NUMERIC",
"AMOUNT_FAILURES_ALLOWED" : "3",
"GEOFENCING_AUTHENTICATION_DENIED_COUNTRIES" : "",
"ACTIVATION_CODE_ALLOWED_GUESS_PROBABILITY" : "1000",
"ATTESTATION_ANDROID_PLAY_INTEGRITY_MODE" : "OFF",
"APNS_NOTIFICATION_SOUND_ENABLED" : "false",
"CLIENT_DEBUG_DATA_ENABLED_OS_TYPES" : "",
"GEOFENCING_TIMEOUT" : "10000",
"PIN_CODE_LENGTH" : "4",
"GEOFENCING_ACTIVATION_ALLOWED_COUNTRIES" : "",
"ACTIVATION_CODE_LENGTH" : "6",
"PIN_CODE_TYPE" : "NUMERIC",
"GEOFENCING_ACTIVATION_DENIED_COUNTRIES" : "",
"NATIVE_PUSH_ENABLED" : "false",
"RECOVERY_CODE_AMOUNT_FAILURES_ALLOWED" : "3",
"FIREBASE_TIME_TO_LIVE" : "0",
"ATTESTATION_IOS_APP_ATTEST_APP_ID" : "",
"ALLOWED_AUTH_METHODS_FOR_AUTH_AND_ACTIVATE" : "\n DEVICE:PIN\n ",
"LOCK_SCOPE" : "AUTH_METHOD",
"ENABLED_RISK_DATA" : ""
}
}, {
"app_id" : "Application-2",
"uuid" : "4f0ae676-dc1d-4d34-bc60-a4eab77d38b8",
"apns_uuid" : "baaa7d9f-ff29-4517-bf36-6dc2241f7e37",
"app_name" : "Application Name 2",
"status" : "ENABLED",
"configuration" : {
"CLIENT_REQUEST_TIME_VALIDATION_THRESHOLD" : "300000",
"RECOVERY_CODE_MIN_LENGTH" : "6",
"GEOFENCING_ACTIVATION_MODE" : "OFF",
"ATTESTATION_IOS_APP_ATTEST_TIMEOUT" : "20000",
"ATTESTATION_IOS_APP_ATTEST_ENVIRONMENT" : "PRODUCTION",
"RECOVERY_CODE_MAX_LENGTH" : "50",
"ALLOWED_AUTH_METHODS" : "\n DEVICE,\n DEVICE:PIN\n ",
"HWKEY_VALIDATION_STRATEGY" : "SUPPORTED",
"GEOFENCING_AUTHENTICATION_ALLOWED_CONTINENTS" : "",
"MAXIMUM_SESSION_EXPIRY" : "300000",
"SESSION_EXPIRY" : "300000",
"RECOVERY_ENABLED" : "false",
"RECOVERY_CODE_FORMAT" : "NUMERIC",
"MAX_PIN_CODE_LENGTH" : "4",
"TOKEN_TYPE" : "SAML2",
"AUTHORIZATION_TOKEN_TIMEOUT" : "300000",
"GEOFENCING_AUTHENTICATION_ALLOWED_COUNTRIES" : "",
"URL_SCHEME" : "encap",
"CLIENT_ONLY_ALLOWED" : "true",
"GEOFENCING_ACTIVATION_ALLOWED_CONTINENTS" : "",
"ATTESTATION_IOS_APP_ATTEST_MODE" : "OFF",
"ATTESTATION_ANDROID_SAFETYNET_MODE" : "OFF",
"APN_EXPIRY" : "86400000",
"APNS_TIME_SENSITIVE_INTERRUPTION_LEVEL_ENABLED" : "false",
"GEOFENCING_AUTHENTICATION_MODE" : "OFF",
"APN_CONFIG" : "PRODUCTION",
"CLIENT_REQUEST_TIME_VALIDATION_ENABLED" : "true",
"ACTIVATION_CODE_TYPE" : "NUMERIC",
"AMOUNT_FAILURES_ALLOWED" : "3",
"GEOFENCING_AUTHENTICATION_DENIED_COUNTRIES" : "",
"ACTIVATION_CODE_ALLOWED_GUESS_PROBABILITY" : "1000",
"ATTESTATION_ANDROID_PLAY_INTEGRITY_MODE" : "OFF",
"APNS_NOTIFICATION_SOUND_ENABLED" : "false",
"CLIENT_DEBUG_DATA_ENABLED_OS_TYPES" : "",
"GEOFENCING_TIMEOUT" : "10000",
"PIN_CODE_LENGTH" : "4",
"GEOFENCING_ACTIVATION_ALLOWED_COUNTRIES" : "",
"ACTIVATION_CODE_LENGTH" : "6",
"PIN_CODE_TYPE" : "NUMERIC",
"GEOFENCING_ACTIVATION_DENIED_COUNTRIES" : "",
"NATIVE_PUSH_ENABLED" : "false",
"RECOVERY_CODE_AMOUNT_FAILURES_ALLOWED" : "3",
"FIREBASE_TIME_TO_LIVE" : "0",
"ATTESTATION_IOS_APP_ATTEST_APP_ID" : "",
"ALLOWED_AUTH_METHODS_FOR_AUTH_AND_ACTIVATE" : "\n DEVICE:PIN\n ",
"LOCK_SCOPE" : "AUTH_METHOD",
"ENABLED_RISK_DATA" : ""
}
} ]
}
4.2.6. Update application configuration
PATCH /api/admin/v1/appconfig/{uuid}
This patch API follows the RFC 7396 JSON Merge Patch standard.
The request body should only include fields that are to be updated.
To remove a field, set the value in the key value pair to null
.
Absence of a key will cause no changes to that field value.
Arguments
Path | Type | Required | Description |
---|---|---|---|
|
|
No |
The status of the application configuration. ENABLED, DISABLED. |
|
|
No |
The UUID of the APNs push certificate or token used. Required if NATIVE_PUSH_ENABLED is true. |
|
|
No |
The human readable name for the application configuration |
|
|
No |
The application configuration parameters. Parameters follow the JSON Merge Patch standard. Absent parameters will be unaffected. |
Returns
Returns an application configuration object if request is processed successfully. Returns an error object if something goes wrong.
Example request
$ curl 'http://localhost:8080/api/admin/v1/appconfig/12269bd0-f124-4153-8ea7-9ba927fd46c8' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X PATCH \
-H 'Content-Type: application/merge-patch+json' \
-d '{
"apns_uuid" : "bb53e278-1e0a-4a03-b594-315c8f49ecd9",
"app_name" : "New Application Name",
"status" : "DISABLED",
"configuration" : {
"CLIENT_ONLY_ALLOWED" : "false",
"ATTESTATION_IOS_APP_ATTEST_TIMEOUT" : null
}
}'
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 9f685d6f-c73b-40ad-828c-e4fbb2677d65
Content-Type: application/json
Content-Length: 2294
{
"app_id" : "some-new-app",
"uuid" : "12269bd0-f124-4153-8ea7-9ba927fd46c8",
"apns_uuid" : "bb53e278-1e0a-4a03-b594-315c8f49ecd9",
"app_name" : "New Application Name",
"status" : "DISABLED",
"configuration" : {
"CLIENT_REQUEST_TIME_VALIDATION_THRESHOLD" : "300000",
"RECOVERY_CODE_MIN_LENGTH" : "6",
"GEOFENCING_ACTIVATION_MODE" : "OFF",
"ATTESTATION_IOS_APP_ATTEST_ENVIRONMENT" : "PRODUCTION",
"RECOVERY_CODE_MAX_LENGTH" : "50",
"ALLOWED_AUTH_METHODS" : "\n DEVICE,\n DEVICE:PIN\n ",
"HWKEY_VALIDATION_STRATEGY" : "SUPPORTED",
"GEOFENCING_AUTHENTICATION_ALLOWED_CONTINENTS" : "",
"MAXIMUM_SESSION_EXPIRY" : "300000",
"SESSION_EXPIRY" : "300000",
"RECOVERY_ENABLED" : "false",
"RECOVERY_CODE_FORMAT" : "NUMERIC",
"MAX_PIN_CODE_LENGTH" : "4",
"TOKEN_TYPE" : "SAML2",
"AUTHORIZATION_TOKEN_TIMEOUT" : "300000",
"GEOFENCING_AUTHENTICATION_ALLOWED_COUNTRIES" : "",
"URL_SCHEME" : "encap",
"CLIENT_ONLY_ALLOWED" : "false",
"GEOFENCING_ACTIVATION_ALLOWED_CONTINENTS" : "",
"ATTESTATION_IOS_APP_ATTEST_MODE" : "OFF",
"ATTESTATION_ANDROID_SAFETYNET_MODE" : "OFF",
"APN_EXPIRY" : "86400000",
"APNS_TIME_SENSITIVE_INTERRUPTION_LEVEL_ENABLED" : "false",
"GEOFENCING_AUTHENTICATION_MODE" : "OFF",
"APN_CONFIG" : "PRODUCTION",
"CLIENT_REQUEST_TIME_VALIDATION_ENABLED" : "true",
"ACTIVATION_CODE_TYPE" : "NUMERIC",
"AMOUNT_FAILURES_ALLOWED" : "3",
"GEOFENCING_AUTHENTICATION_DENIED_COUNTRIES" : "",
"ACTIVATION_CODE_ALLOWED_GUESS_PROBABILITY" : "1000",
"ATTESTATION_ANDROID_PLAY_INTEGRITY_MODE" : "OFF",
"APNS_NOTIFICATION_SOUND_ENABLED" : "false",
"CLIENT_DEBUG_DATA_ENABLED_OS_TYPES" : "",
"GEOFENCING_TIMEOUT" : "10000",
"PIN_CODE_LENGTH" : "4",
"GEOFENCING_ACTIVATION_ALLOWED_COUNTRIES" : "",
"ACTIVATION_CODE_LENGTH" : "6",
"PIN_CODE_TYPE" : "NUMERIC",
"GEOFENCING_ACTIVATION_DENIED_COUNTRIES" : "",
"NATIVE_PUSH_ENABLED" : "false",
"RECOVERY_CODE_AMOUNT_FAILURES_ALLOWED" : "3",
"FIREBASE_TIME_TO_LIVE" : "0",
"ATTESTATION_IOS_APP_ATTEST_APP_ID" : "",
"ALLOWED_AUTH_METHODS_FOR_AUTH_AND_ACTIVATE" : "\n DEVICE:PIN\n ",
"LOCK_SCOPE" : "AUTH_METHOD",
"ENABLED_RISK_DATA" : ""
}
}
4.2.7. Delete application configuration
DELETE /api/admin/v1/appconfig/{uuid}
Removes an application configuration with the uuid.
Example request
$ curl 'http://localhost:8080/api/admin/v1/appconfig/3cf5fdc5-201a-4af6-9a9e-04195572d0fc' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X DELETE \
-H 'Content-Type: application/json'
Example response
HTTP/1.1 202 Accepted
X-Correlation-UUID: 7fedc8b6-e361-489b-a5cd-9fe039e534fa
4.3. APNs Certificate
An API to create and retrieve APNs Certificates. Once a certificate is associated with an application configuration, that application will then use the APNs Certificate to send push notifications to Apple devices. Encap supports APNs tokens, for details on how configure an APNs token please see the Encap Administration Manual.
4.3.1. The APNs object
Field | Type | Description |
---|---|---|
|
|
The UUID for the APNS certificate |
|
|
The name of the APNS certificate |
|
|
The SHA1 hash of the APNS certificate |
|
|
The SHA256 hash of the APNS certificate |
|
|
The description of the APNS certificate |
|
|
The start date of the certificate validity period |
|
|
The end date of the certificate validity period |
|
|
The subject distinguished name from the certificate |
|
|
The issuer distinguished name from the certificate |
|
|
The name/id of the creator of the APNS certificate |
|
|
The date for when the APNS certificate was created. Returned in pattern yyyy-MM-dd’T’HH:mm:ss.SSS’UTC' |
4.3.2. Create APNs Certificate
POST /api/admin/v1/apns
Creates a new APNs Certificate with the provided values
Arguments
Path | Type | Required | Description |
---|---|---|---|
|
|
Yes |
The name of the APNS certificate |
|
|
No |
The description of the APNS certificate |
|
|
Yes |
The Base64 encoded string of the APNS certificate bytes |
|
|
Yes |
The password associated with the APNS certificate |
Returns
Returns an apns certificate object if the request was processed successfully, and returns an error otherwise.
Example request
$ curl 'http://localhost:8080/api/admin/v1/apns' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X POST \
-H 'Content-Type: application/json' \
-d '{
"name" : "test-cert-name",
"description" : "test-cert-description",
"apns_certificate_b64" : "somelongb64encodedstring",
"password" : "test-cert-password"
}'
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 524456de-4689-4767-8539-30d07f081de7
Content-Type: application/json
Content-Length: 739
{
"id" : "681d6d77-1c57-4e72-b4c4-bdf02d49a87c",
"name" : "test-cert-name",
"description" : "test-cert-description",
"created_by" : "someuser",
"created_on" : "2025-03-17T16:58:05.902UTC",
"sha1_fingerprint" : "d0:5b:e1:40:6e:78:5d:10:cf:a8:67:c5:5a:02:5b:7f:de:7d:93:d2",
"sha256_fingerprint" : "c3:64:fb:f9:a8:46:04:5c:ed:ef:bc:1c:78:00:cb:b7:6d:67:64:50:16:cd:3e:5e:69:04:30:5c:59:ff:02:4a",
"valid_from" : "2025-01-31T12:26:56.000UTC",
"valid_until" : "2026-03-02T12:26:55.000UTC",
"subject" : "C=NO,O=Signicat AS,OU=Y2Y8VXX8PM,CN=Apple Push Services: com.signicat.encap.TestApp,UID=com.signicat.encap.TestApp",
"issuer" : "C=US,O=Apple Inc.,OU=G4,CN=Apple Worldwide Developer Relations Certification Authority"
}
4.3.3. Get APNs Certificate details
GET /api/admin/v1/apns/{apnsId}
Get meta data for the APNs Certificate
Returns
Returns a apns certificate object if a valid identifier was provided, and returns an error otherwise.
Example request
$ curl 'http://localhost:8080/api/admin/v1/apns/681d6d77-1c57-4e72-b4c4-bdf02d49a87c' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X GET
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 96521baa-ea5b-40fd-ac74-76467c0dec50
Content-Type: application/json
Content-Length: 739
{
"id" : "681d6d77-1c57-4e72-b4c4-bdf02d49a87c",
"name" : "test-cert-name",
"description" : "test-cert-description",
"created_by" : "someuser",
"created_on" : "2025-03-17T16:58:06.653UTC",
"sha1_fingerprint" : "d0:5b:e1:40:6e:78:5d:10:cf:a8:67:c5:5a:02:5b:7f:de:7d:93:d2",
"sha256_fingerprint" : "c3:64:fb:f9:a8:46:04:5c:ed:ef:bc:1c:78:00:cb:b7:6d:67:64:50:16:cd:3e:5e:69:04:30:5c:59:ff:02:4a",
"valid_from" : "2025-01-31T12:26:56.000UTC",
"valid_until" : "2026-03-02T12:26:55.000UTC",
"subject" : "C=NO,O=Signicat AS,OU=Y2Y8VXX8PM,CN=Apple Push Services: com.signicat.encap.TestApp,UID=com.signicat.encap.TestApp",
"issuer" : "C=US,O=Apple Inc.,OU=G4,CN=Apple Worldwide Developer Relations Certification Authority"
}
4.3.4. Get all APNs Certificates
GET /api/admin/v1/apns
Returns a pagination object with a list of apns certificate objects that belongs to your organization.
Example request
$ curl 'http://localhost:8080/api/admin/v1/apns?limit=3&offset=0f29c6c5-1ba7-4b7a-9530-ad549822254e' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X GET
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 8ca387a1-f373-4f53-8c2a-728788b82422
Content-Type: application/json
Content-Length: 2547
{
"previous" : "/admin/v1/apns?limit=3&offset=00e7071a-be0d-458b-afad-26645bb99ed9",
"next" : "/admin/v1/apns?limit=3&offset=68492048-a47e-4b4d-a188-bef6099a20a7",
"offset" : "0f29c6c5-1ba7-4b7a-9530-ad549822254e",
"limit" : 3,
"items" : [ {
"id" : "1aa5cf91-cf82-4d00-9acc-f76f4caa12c0",
"name" : "test-cert-name",
"description" : "test-cert-description",
"created_by" : "someuser",
"created_on" : "2025-03-17T16:58:06.140UTC",
"sha1_fingerprint" : "d0:5b:e1:40:6e:78:5d:10:cf:a8:67:c5:5a:02:5b:7f:de:7d:93:d2",
"sha256_fingerprint" : "c3:64:fb:f9:a8:46:04:5c:ed:ef:bc:1c:78:00:cb:b7:6d:67:64:50:16:cd:3e:5e:69:04:30:5c:59:ff:02:4a",
"valid_from" : "2025-01-31T12:26:56.000UTC",
"valid_until" : "2026-03-02T12:26:55.000UTC",
"subject" : "C=NO,O=Signicat AS,OU=Y2Y8VXX8PM,CN=Apple Push Services: com.signicat.encap.TestApp,UID=com.signicat.encap.TestApp",
"issuer" : "C=US,O=Apple Inc.,OU=G4,CN=Apple Worldwide Developer Relations Certification Authority"
}, {
"id" : "9e876798-a11d-4bd0-bfa4-edc4f92ebd89",
"name" : "test-cert-name",
"description" : "test-cert-description",
"created_by" : "someuser",
"created_on" : "2025-03-17T16:58:06.195UTC",
"sha1_fingerprint" : "d0:5b:e1:40:6e:78:5d:10:cf:a8:67:c5:5a:02:5b:7f:de:7d:93:d2",
"sha256_fingerprint" : "c3:64:fb:f9:a8:46:04:5c:ed:ef:bc:1c:78:00:cb:b7:6d:67:64:50:16:cd:3e:5e:69:04:30:5c:59:ff:02:4a",
"valid_from" : "2025-01-31T12:26:56.000UTC",
"valid_until" : "2026-03-02T12:26:55.000UTC",
"subject" : "C=NO,O=Signicat AS,OU=Y2Y8VXX8PM,CN=Apple Push Services: com.signicat.encap.TestApp,UID=com.signicat.encap.TestApp",
"issuer" : "C=US,O=Apple Inc.,OU=G4,CN=Apple Worldwide Developer Relations Certification Authority"
}, {
"id" : "68da13a5-077a-4d9a-9bd3-568cc9f1f338",
"name" : "test-cert-name",
"description" : "test-cert-description",
"created_by" : "someuser",
"created_on" : "2025-03-17T16:58:06.248UTC",
"sha1_fingerprint" : "d0:5b:e1:40:6e:78:5d:10:cf:a8:67:c5:5a:02:5b:7f:de:7d:93:d2",
"sha256_fingerprint" : "c3:64:fb:f9:a8:46:04:5c:ed:ef:bc:1c:78:00:cb:b7:6d:67:64:50:16:cd:3e:5e:69:04:30:5c:59:ff:02:4a",
"valid_from" : "2025-01-31T12:26:56.000UTC",
"valid_until" : "2026-03-02T12:26:55.000UTC",
"subject" : "C=NO,O=Signicat AS,OU=Y2Y8VXX8PM,CN=Apple Push Services: com.signicat.encap.TestApp,UID=com.signicat.encap.TestApp",
"issuer" : "C=US,O=Apple Inc.,OU=G4,CN=Apple Worldwide Developer Relations Certification Authority"
} ]
}
4.3.5. Delete APNs Certificate
DELETE /api/admin/v1/apns/{apnsId}
Deletes the APNs certificate with the apnsId
Example request
$ curl 'http://localhost:8080/api/admin/v1/apns/e8dd3024-3f05-45b7-991d-b3e6c65279a2' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X DELETE
Example response
HTTP/1.1 204 No Content
X-Correlation-UUID: 768e6b9e-7210-43b2-aff3-cc6813509bb1
4.4. APNs Provider Token
An API to add and retrieve APNs provider tokens.
4.4.1. The APNs provider token object
Field | Type | Description |
---|---|---|
|
|
The UUID for the APNs token |
|
|
The name of the APNs token |
|
|
The SHA1 hash of the APNs token |
|
|
The SHA256 hash of the APNs token |
|
|
The description of the APNs token |
|
|
Key ID of the APNs token |
|
|
Team ID of Apple Developer Account |
|
|
The name/id of the creator of the APNs token |
|
|
The date for when the APNs token was created. Returned in pattern yyyy-MM-dd’T’HH:mm:ss.SSS’UTC' |
4.4.2. Add APNs provider token
POST /api/admin/v1/apnstokens
Adds a new APNs provider token with the provided values (from the Apple Developer Portal).
Arguments
Path | Type | Required | Description |
---|---|---|---|
|
|
Yes |
The name of the APNs token |
|
|
No |
The description of the APNs token |
|
|
Yes |
The Base64 encoded string of APNs token private key |
|
|
Yes |
Key ID of the APNs token |
|
|
Yes |
Team ID of Apple Developer Account |
Returns
Returns an APNs provider token object if the request was processed successfully, and returns an error otherwise.
Example request
$ curl 'http://localhost:8080/api/admin/v1/apnstokens' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X POST \
-H 'Content-Type: application/json' \
-d '{
"name" : "test-token-name",
"description" : "test-token-description",
"private_key_b64" : "privateKeyString",
"key_id" : "key-id",
"team_id" : "team-id"
}'
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 6ed8942f-c1a1-4475-beaa-07827764a6d9
Content-Type: application/json
Content-Length: 467
{
"id" : "4d985445-537d-4e31-823e-1bc8c2f6c849",
"name" : "test-cert-name",
"description" : "test-cert-description",
"created_by" : "someuser",
"created_on" : "2025-03-17T16:58:05.682UTC",
"sha1_fingerprint" : "aa:6b:bb:f2:e3:5e:8f:91:5b:db:11:0a:27:45:0d:59:61:73:3d:6e",
"sha256_fingerprint" : "29:31:57:72:39:ec:78:84:ad:30:68:1a:e3:dc:5a:19:a5:4b:0c:f5:05:c7:48:56:14:59:28:a0:67:97:18:99",
"key_id" : "test-key-id",
"team_id" : "test-team-id"
}
4.4.3. Get APNs provider token details
GET /api/admin/v1/apnstokens/{apnsTokenId}
Get metadata for an APNs provider token
Returns
Returns a APNs provider token object if a valid identifier was provided, and returns an error otherwise.
Example request
$ curl 'http://localhost:8080/api/admin/v1/apnstokens/4d985445-537d-4e31-823e-1bc8c2f6c849' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X GET
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 666639cc-25d7-4281-9022-18fb0f79ca76
Content-Type: application/json
Content-Length: 467
{
"id" : "4d985445-537d-4e31-823e-1bc8c2f6c849",
"name" : "test-cert-name",
"description" : "test-cert-description",
"created_by" : "someuser",
"created_on" : "2025-03-17T16:58:06.183UTC",
"sha1_fingerprint" : "aa:6b:bb:f2:e3:5e:8f:91:5b:db:11:0a:27:45:0d:59:61:73:3d:6e",
"sha256_fingerprint" : "29:31:57:72:39:ec:78:84:ad:30:68:1a:e3:dc:5a:19:a5:4b:0c:f5:05:c7:48:56:14:59:28:a0:67:97:18:99",
"key_id" : "test-key-id",
"team_id" : "test-team-id"
}
4.4.4. Get all APNs provider tokens
GET /api/admin/v1/apnstokens
Returns a pagination object with a list of APNs provider token objects that belongs to your organization.
Example request
$ curl 'http://localhost:8080/api/admin/v1/apnstokens?limit=3&offset=138a0aa0-a0f9-44ef-b076-3f37520ebe2f' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X GET
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 39c9ffae-f99c-464d-b516-d7f495cabcf2
Content-Type: application/json
Content-Length: 1731
{
"previous" : "/admin/v1/apnstokens?limit=3&offset=af60bc6c-4170-4634-b8ff-582d63848406",
"next" : "/admin/v1/apnstokens?limit=3&offset=0c342717-769d-4370-9548-19c5aaa1b924",
"offset" : "138a0aa0-a0f9-44ef-b076-3f37520ebe2f",
"limit" : 3,
"items" : [ {
"id" : "a136eded-c75c-4938-af79-2c0e6e11f998",
"name" : "test-cert-name",
"description" : "test-cert-description",
"created_by" : "someuser",
"created_on" : "2025-03-17T16:58:05.506UTC",
"sha1_fingerprint" : "aa:6b:bb:f2:e3:5e:8f:91:5b:db:11:0a:27:45:0d:59:61:73:3d:6e",
"sha256_fingerprint" : "29:31:57:72:39:ec:78:84:ad:30:68:1a:e3:dc:5a:19:a5:4b:0c:f5:05:c7:48:56:14:59:28:a0:67:97:18:99",
"key_id" : "test-key-id",
"team_id" : "test-team-id"
}, {
"id" : "5314c81c-0340-4dbb-ae51-ef34cef00b61",
"name" : "test-cert-name",
"description" : "test-cert-description",
"created_by" : "someuser",
"created_on" : "2025-03-17T16:58:05.507UTC",
"sha1_fingerprint" : "aa:6b:bb:f2:e3:5e:8f:91:5b:db:11:0a:27:45:0d:59:61:73:3d:6e",
"sha256_fingerprint" : "29:31:57:72:39:ec:78:84:ad:30:68:1a:e3:dc:5a:19:a5:4b:0c:f5:05:c7:48:56:14:59:28:a0:67:97:18:99",
"key_id" : "test-key-id",
"team_id" : "test-team-id"
}, {
"id" : "46c28b42-d428-4fe0-bdac-5130a12cb593",
"name" : "test-cert-name",
"description" : "test-cert-description",
"created_by" : "someuser",
"created_on" : "2025-03-17T16:58:05.507UTC",
"sha1_fingerprint" : "aa:6b:bb:f2:e3:5e:8f:91:5b:db:11:0a:27:45:0d:59:61:73:3d:6e",
"sha256_fingerprint" : "29:31:57:72:39:ec:78:84:ad:30:68:1a:e3:dc:5a:19:a5:4b:0c:f5:05:c7:48:56:14:59:28:a0:67:97:18:99",
"key_id" : "test-key-id",
"team_id" : "test-team-id"
} ]
}
4.4.5. Delete APNs provider token
DELETE /api/admin/v1/apnstokens/{apnsTokenId}
Deletes the APNs provider token with the apnsTokenId
Example request
$ curl 'http://localhost:8080/api/admin/v1/apnstokens/61542b51-3798-4d72-a496-6afc1166b1f5' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X DELETE
Example response
HTTP/1.1 204 No Content
X-Correlation-UUID: 21ce335a-0aef-4845-a665-2d5e927d1cc5
4.5. API Key
An API to manage API keys. API keys are used to authenticate on all Encap REST APIs.
4.5.1. The API key object
Field | Type | Description |
---|---|---|
|
|
The API key ID, UUID, for the key. |
|
|
The API key secret. The secret is only part of the response when a API key is created, it is not returned when you fetch an API key |
|
|
The description for the API key |
|
|
The name/id of the creator of the API key |
|
|
The date for when the API key was created. Returned in pattern yyyy-MM-dd’T’HH:mm:ss.SSS’UTC' |
4.5.2. Create API Key
POST /api/admin/v1/api-keys
Create a new API key
Arguments
Path | Type | Required | Description |
---|---|---|---|
|
|
No |
The description for the API key |
Returns
Example request
$ curl 'http://localhost:8080/api/admin/v1/api-keys' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X POST \
-H 'Content-Type: application/json' \
-d '{
"description" : "Key for client A"
}'
Example response
HTTP/1.1 201 Created
X-Correlation-UUID: 7945be11-c898-45f1-a9fb-f434892c4f88
Location: /admin/v1/api-keys/d85e5dfc-700f-4d2a-8c97-bc556d3cf625
Content-Type: application/json
Content-Length: 250
{
"api_key_id" : "d85e5dfc-700f-4d2a-8c97-bc556d3cf625",
"created_on" : "2025-03-17T16:58:03.024UTC",
"created_by" : "85d8884c-c1f2-4bd1-b46c-0f0af07ae77a",
"description" : "Key for client A",
"api_key_secret" : "ltXaQjVJYnTiI/3xmBdfrg=="
}
4.5.3. Get API key details
GET /api/admin/v1/api-keys/{api_key_id}
Get details for an API key.
Returns
Example request
$ curl 'http://localhost:8080/api/admin/v1/api-keys/d85e5dfc-700f-4d2a-8c97-bc556d3cf625' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X GET
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 6aad8497-20cc-46cf-a25c-416b5b537c67
Content-Type: application/json
Content-Length: 201
{
"api_key_id" : "d85e5dfc-700f-4d2a-8c97-bc556d3cf625",
"created_on" : "2025-03-17T16:58:03.533UTC",
"created_by" : "85d8884c-c1f2-4bd1-b46c-0f0af07ae77a",
"description" : "Key for client A"
}
4.5.4. Get all API keys
GET /api/admin/v1/api-keys
Returns a pagination object with a list of API key objects that belongs your organization.
Example request
$ curl 'http://localhost:8080/api/admin/v1/api-keys/?limit=3&offset=d5c0018d-e15f-4ed9-915c-f48e57ce911c' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X GET
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: b563dced-bad2-47e4-b705-57a54c7395c9
Content-Type: application/json
Content-Length: 899
{
"previous" : "/admin/v1/api-keys?limit=3&offset=0e879f2a-6fac-4667-8872-cedf833353d3",
"next" : "/admin/v1/api-keys?limit=3&offset=09e0d44d-1939-4d68-b56a-e2117475fa51",
"offset" : "d5c0018d-e15f-4ed9-915c-f48e57ce911c",
"limit" : 3,
"items" : [ {
"api_key_id" : "452c4294-2d56-465d-ae42-6f3654c3e759",
"created_on" : "2025-03-17T16:58:03.697UTC",
"created_by" : "85d8884c-c1f2-4bd1-b46c-0f0af07ae77a",
"description" : "Key for client A"
}, {
"api_key_id" : "9cc1f0b9-0ec4-47de-b2aa-b76ad61c5715",
"created_on" : "2025-03-17T16:58:03.697UTC",
"created_by" : "85d8884c-c1f2-4bd1-b46c-0f0af07ae77a",
"description" : "Key for client A"
}, {
"api_key_id" : "0e95d187-fc03-407d-8411-2a8fcab058f8",
"created_on" : "2025-03-17T16:58:03.697UTC",
"created_by" : "85d8884c-c1f2-4bd1-b46c-0f0af07ae77a",
"description" : "Key for client A"
} ]
}
4.5.5. Delete API key
DELETE /api/admin/v1/api-keys/{api_key_id}
Deletes the API key with the specified id
Example request
$ curl 'http://localhost:8080/api/admin/v1/api-keys/d85e5dfc-700f-4d2a-8c97-bc556d3cf625' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X DELETE
Example response
HTTP/1.1 204 No Content
X-Correlation-UUID: dd8dde85-0bdb-48d7-b03b-200c82f74b93
4.6. End-to-End Key
End-to-End keys are used by Encap to create an additional encryption layer between the Encap Server and the SDK. This API is used to create and manage the End-to-End encryption keys used in this layer. Each organisation needs at least one End-to-End Key, but preferably you should have one key per application.
4.6.1. The End-to-End Key Object
Field | Type | Description |
---|---|---|
|
|
The UUID for the End-To-End key |
|
|
Name for the End-To-End key |
|
|
Description for the End-To-End key |
|
|
The name/id of the creator of the End-To-End key |
|
|
The date for when the End-To-End key was created. Returned in pattern yyyy-MM-dd’T’HH:mm:ss.SSS’UTC' |
|
|
The SHA256 hash of the End-To-End key certificate |
|
|
Base64 encoded End-To-End key certificate |
|
|
The UUID for the organization that owns this End-To-End key |
|
|
Status of the End-To-End key. Possible values are ENABLED and DISABLED |
4.6.2. Create End-to-End Key
POST /api/admin/v1/e2e-keys
Create a new End-to-End Key. A new End-to-End key pair is generated by the server and the public key is sent back in the response.
Arguments
Path | Type | Required | Description |
---|---|---|---|
|
|
Yes |
The name of the End-To-End key |
|
|
No |
Description for the End-To-End key |
Returns
Returns an End-to-End Key object if the request was processed successfully, and returns an error otherwise.
Example request
$ curl 'http://localhost:8080/api/admin/v1/e2e-keys' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X POST \
-H 'Content-Type: application/json' \
-d '{
"name" : "test-e2e-key-name",
"description" : "test-e2e-key-description"
}'
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 8ebaa082-d8c6-4ee0-8d0b-06aa2a4662fb
Content-Type: application/json
Content-Length: 551
{
"id" : "9999e032-94cf-4ea4-96ac-ab665018d10a",
"name" : "test-e2e-cert-name",
"description" : "test-e2e-cert-description",
"created_by" : "someuser",
"created_on" : "2022-11-30T18:35:24.000UTC",
"sha256_fingerprint" : "71:04:a9:dc:e4:3c:23:fe:d8:d3:8f:79:f0:d7:1a:2e:35:7d:c6:de:6d:fd:19:9e:07:74:23:35:82:83:37:22",
"public_key" : "MFIwEAYHKoZIzj0CAQYFK4EEABoDPgAEAfdEY0BYY8yINZaHED77apY3/nZK3HZSnkUOrqUVAUcHe+RGt/iitJy8CGQmMjPA/gql5mREyiAPAPg2",
"organization_id" : "e30ebcb4-3f19-4ae7-aa10-01d19c08a05c",
"status" : "DISABLED"
}
4.6.3. Get End-to-End Key details
GET /api/admin/v1/e2e-keys/{e2eKeyId}
Get details for an End-to-End Key.
Returns
Returns a End-to-End Key object if a valid identifier was provided, and returns an error otherwise.
Example request
$ curl 'http://localhost:8080/api/admin/v1/e2e-keys/9999e032-94cf-4ea4-96ac-ab665018d10a' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X GET
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 91097517-c609-4688-a154-4cfbd0b01bb2
Content-Type: application/json
Content-Length: 551
{
"id" : "9999e032-94cf-4ea4-96ac-ab665018d10a",
"name" : "test-e2e-cert-name",
"description" : "test-e2e-cert-description",
"created_by" : "someuser",
"created_on" : "2022-11-30T18:35:24.000UTC",
"sha256_fingerprint" : "71:04:a9:dc:e4:3c:23:fe:d8:d3:8f:79:f0:d7:1a:2e:35:7d:c6:de:6d:fd:19:9e:07:74:23:35:82:83:37:22",
"public_key" : "MFIwEAYHKoZIzj0CAQYFK4EEABoDPgAEAfdEY0BYY8yINZaHED77apY3/nZK3HZSnkUOrqUVAUcHe+RGt/iitJy8CGQmMjPA/gql5mREyiAPAPg2",
"organization_id" : "a52e514c-440f-4038-a3a7-616ec50fe078",
"status" : "DISABLED"
}
4.6.4. Get all End-to-End Keys
GET /api/admin/v1/e2e-keys
Returns a pagination object with a list of End-to-End Key objects that belongs your organization.
Example request
$ curl 'http://localhost:8080/api/admin/v1/e2e-keys?limit=3&offset=87223d04-260f-44e6-8c9e-fd4af0e71f0c' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X GET
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: bacc94f2-dcd4-411a-b524-57b455dcb26b
Content-Type: application/json
Content-Length: 1979
{
"previous" : "/admin/v1/e2e-keys?limit=3&offset=69298b3b-4c4a-4f55-930c-7985d2f95fcb",
"next" : "/admin/v1/e2e-keys?limit=3&offset=793a91e8-c66d-4c7f-9ee1-6d2d8fef183a",
"offset" : "87223d04-260f-44e6-8c9e-fd4af0e71f0c",
"limit" : 3,
"items" : [ {
"id" : "45ef6254-e8e8-4692-8e2b-4147dbce1afa",
"name" : "test-e2e-cert-name",
"description" : "test-e2e-cert-description",
"created_by" : "someuser",
"created_on" : "2022-11-30T18:35:24.000UTC",
"sha256_fingerprint" : "71:04:a9:dc:e4:3c:23:fe:d8:d3:8f:79:f0:d7:1a:2e:35:7d:c6:de:6d:fd:19:9e:07:74:23:35:82:83:37:22",
"public_key" : "MFIwEAYHKoZIzj0CAQYFK4EEABoDPgAEAfdEY0BYY8yINZaHED77apY3/nZK3HZSnkUOrqUVAUcHe+RGt/iitJy8CGQmMjPA/gql5mREyiAPAPg2",
"organization_id" : "556c0805-6477-4706-8945-4af3ac41d184",
"status" : "DISABLED"
}, {
"id" : "3e6281c4-755e-4289-b144-9cb8d1b4a2e1",
"name" : "test-e2e-cert-name",
"description" : "test-e2e-cert-description",
"created_by" : "someuser",
"created_on" : "2022-11-30T18:35:24.000UTC",
"sha256_fingerprint" : "71:04:a9:dc:e4:3c:23:fe:d8:d3:8f:79:f0:d7:1a:2e:35:7d:c6:de:6d:fd:19:9e:07:74:23:35:82:83:37:22",
"public_key" : "MFIwEAYHKoZIzj0CAQYFK4EEABoDPgAEAfdEY0BYY8yINZaHED77apY3/nZK3HZSnkUOrqUVAUcHe+RGt/iitJy8CGQmMjPA/gql5mREyiAPAPg2",
"organization_id" : "7ea45c46-1776-4443-a2d7-55d27afacd62",
"status" : "DISABLED"
}, {
"id" : "7a579e70-811a-44b3-a926-41f56e58fc90",
"name" : "test-e2e-cert-name",
"description" : "test-e2e-cert-description",
"created_by" : "someuser",
"created_on" : "2022-11-30T18:35:24.000UTC",
"sha256_fingerprint" : "71:04:a9:dc:e4:3c:23:fe:d8:d3:8f:79:f0:d7:1a:2e:35:7d:c6:de:6d:fd:19:9e:07:74:23:35:82:83:37:22",
"public_key" : "MFIwEAYHKoZIzj0CAQYFK4EEABoDPgAEAfdEY0BYY8yINZaHED77apY3/nZK3HZSnkUOrqUVAUcHe+RGt/iitJy8CGQmMjPA/gql5mREyiAPAPg2",
"organization_id" : "6b27be91-baa2-4e4b-a350-832028f035ab",
"status" : "DISABLED"
} ]
}
4.6.5. Update status of End-to-End Key
PUT /api/admin/v1/e2e-keys/{e2eKeyId}/status
Changing the status of the key. Changing status to DISABLED means that it will no longer be usable for client requests.
Arguments
Path | Type | Required | Description |
---|---|---|---|
|
|
Yes |
Status to set for the End-To-End key. Possible values are ENABLED and DISABLED |
Example request
$ curl 'http://localhost:8080/api/admin/v1/e2e-keys/9999e032-94cf-4ea4-96ac-ab665018d10a/status' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X PUT \
-H 'Content-Type: application/json' \
-d '{
"status" : "DISABLED"
}'
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: b87c5fcf-ed23-49e2-a08b-7d26963b252e
Content-Type: application/json
Content-Length: 27
{
"status" : "DISABLED"
}
4.6.6. Delete End-to-End Key
DELETE /api/admin/v1/e2e-keys/{e2eKeyId}
Deletes the End-to-End Key with the specified id. You can only delete unused keys (having status set to DISABLED).
Example request
$ curl 'http://localhost:8080/api/admin/v1/e2e-keys/9999e032-94cf-4ea4-96ac-ab665018d10a' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X DELETE
Example response
HTTP/1.1 204 No Content
X-Correlation-UUID: 0b348230-ca32-4d80-a3cc-52fd0ef0691a
5. Statistics API
5.1. Encap client versions
An API for extracting statistics about the Encap client version for the devices registered in Encap. The statistics result will provide information on the amount of registrations that has been active within a given time interval, grouped by the Encap version of the client.
5.1.1. Usage
The version statistics extraction is a two-step progress because the actual data-extraction might take some time given a large dataset.
The first call, a POST request, will creates a new asynchrounous job for extracting the version statistics, the request returns an UUID for the version statistics result. This UUID is used in the second step. The second call, a GET request, gets the result of version statistics. If you perform a GET request before the extraction job has finished, the response will have the status IN_PROGRESS.
We also provided a cache functionality for the statistic extraction. If you make a new POST request for the same application configuration and the same time interval as a previous request, that has finished within the cache time period, then the server will not create a new job for extracting the same data. But instead return with the status READY and the UUID of the previous version statistics as a reference. To get the complete response perform a GET request for that UUID. If the previous version extraction has not yet finished, then the server will not start a new extraction job regardless of the cache time, but instead return with the status IN_PROGRESS and the UUID of the previous version statistics as a reference. To get the complete response perform a GET request for that UUID.
The cache time has a default value of one hour. You can change the cache time by providing the cluster-property admin.statistics.cachetime
.
This value is an integer of seconds.
5.1.2. The encap client versions object
Field | Type | Description |
---|---|---|
|
|
The id of the statistics record |
|
|
Status of the statistics record. May be IN_PROGRESS, READY or FAILED |
|
|
UUID of the app config the statistics are generated for |
|
|
Registrations used after the number of days from this field will be included in the statistic |
|
|
The time the record was first created |
|
|
Number of devices used in the last amount of days, dictated by last_used, this is split by version |
Field | Type | Description |
---|---|---|
|
|
Encap client version |
|
|
Number of devices on this version |
5.1.3. Request statistics
POST /api/statistics/v1/encap-client-versions
Arguments
Path | Type | Required | Description |
---|---|---|---|
|
|
Yes |
UUID of the app config the statistics are generated for |
|
|
Yes |
Registrations used after the number of days from this field will be included in the statistic |
Example request body
{
"app_config_uuid" : "a8c06f07-a112-42cf-bd1e-da8995f87e52",
"last_used" : 365
}
Returns
Returns an encap client versions object if the request statistics is successfully initiated. Returns an error object if something goes wrong.
Example request
$ curl 'http://localhost:8080/api/statistics/v1/encap-client-versions/' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X POST \
-H 'Content-Type: application/json' \
-d '{
"app_config_uuid" : "a8c06f07-a112-42cf-bd1e-da8995f87e52",
"last_used" : 365
}'
Example response:
HTTP/1.1 202 Accepted
X-Correlation-UUID: 6ad8557c-5de5-4254-ab35-6a20ad30ce63
Content-Type: application/json
Content-Length: 243
{
"uuid" : "7ab4421d-c762-4f6a-9953-35f75786ccdd",
"status" : "IN_PROGRESS",
"app_config_uuid" : "a8c06f07-a112-42cf-bd1e-da8995f87e52",
"last_used" : 365,
"generated_on" : "2025-03-17T16:58:09.146UTC",
"version_statistics" : [ ]
}
5.1.4. Get statistics record
GET /api/statistics/v1/encap-client-versions/{uuid}
Returns
Returns an encap client versions object if a valid identifier was provided, and returns an error otherwise.
Example request
$ curl 'http://localhost:8080/api/statistics/v1/encap-client-versions/7ab4421d-c762-4f6a-9953-35f75786ccdd' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X GET
Example response
HTTP/1.1 200 OK
X-Correlation-UUID: 9bd56828-abd9-44de-9b32-4bb6b294ba08
Content-Type: application/json
Content-Length: 298
{
"uuid" : "7ab4421d-c762-4f6a-9953-35f75786ccdd",
"status" : "READY",
"app_config_uuid" : "a8c06f07-a112-42cf-bd1e-da8995f87e52",
"last_used" : 365,
"generated_on" : "2025-03-17T16:58:08.955UTC",
"version_statistics" : [ {
"encap_client_version" : "3.9.0",
"count" : 10
} ]
}
6. Callback
Encap supports two different service provider callbacks models, On-demand callback and Event callback. On-demand callback is requested for each activation or authentication operation, and provides a full response for the operation. Event callbacks are configured on the application configuration, and supports events for activation, authentication in addition to other events. The callback gives a status of the operation and a reference to the full response. Event callback is more resilient against event delivery issues and has a persistent event storage. It is possible to use both Event callback and On-demand callback in parallel.
6.1. On-demand callback
Callback for device activation and authentication, is used to inform the service provider about the status of a completed activation or authentication. The service provider can then wait for the callback instead of polling the server for status for the ongoing session.
6.1.1. Implementation
The callback endpoint must be implemented by the Service Provider and be reachable from the Encap server.
The endpoint needs to accept POST of the payloads described enrollment callback and here authentication callback.
The Encap server interprets any 2xx response as a success. Any other response will lead to a warning being logged in the server log, but this will have no effect on the status of the ongoing operation.
6.1.2. Usage
If the ´callback_address´ argument is set when creating the authentication object or enrollment, a callback will be sent by the Encap server to this address when the operation is completed (status is SUCCESS or FAILURE).
6.1.3. Important operational behaviour
The callback is performed asynchronously, so the client device will get an immediate response regardless of the availability of the service provider callback endpoint.
In order to monitor the health of the callback service, the operator needs to monitor (and act upon) Encap log messages with WARNING level for failing callbacks. Failure to do so will cause the device to think it is successfully activated or authenticated without the service provider being informed by of the result of the operation.
6.2. Event callback
Event callback reduces the need to poll for status changes. Event callback notifications are sent asynchronously to the service provider for selected events in Encap SCA. Events can also be filtered based on which API that initiated the operation.
6.2.1. Configuration
Event callback global properties
The encap-cluster.properties file contains parameters relevant for the configuration of the Event Callback feature. Cluster wide parameters for polling intervals, retries and timeouts can be configured to control the behaviour of the Event Callback service.
More details about the cluster configuration properties han be found in the Encap Server Administration Manual, chapter "Server config params".
Application configuration parameters
The application configuration parameter EVENT_CALLBACK_EVENTS is used to configure which events and APIs you want to trigger a callback. The EVENT_CALLBACK_URL parameter controls the URL for posting the event-callback objects.
This means that you can have a different set of events/APIs & URL per application config.
More details about the application configuration parameters.
Event types
Event type | Description |
---|---|
|
Device locked (status SUCCESS) |
|
Device unlocked (status SUCCESS) |
|
Device deactivated (status SUCCESS) |
|
Activation session has been completed (status SUCCESS or FAILURE) |
|
Authentication session has been completed (status SUCCESS or FAILURE) |
|
Recovery method has been added to the device (status SUCCESS) |
|
Recovery method has been removed from the device (status SUCCESS) |
|
Device recovery has been performed (status SUCCESS) |
Event APIs
API type | Description |
---|---|
|
Event for operations initiated by the mobile device |
|
Event for operations initiated from the REST API |
6.2.2. Event callback service provider API
Event callback API for notifying the service provider when events occur in Encap.
Encap server performs event-callback to service provider
POST /service-provider/event-callback-url
A http POST will be performed when the application configuration for the device has event callback configured
The event callback object
Path | Type | Required | Description |
---|---|---|---|
|
|
Yes |
Unique event ID. Can be used to identify duplicate callbacks for the same event (rare state) |
|
|
Yes |
The type of event that has occurred. See event-callback-events for possible values |
|
|
Yes |
The UUID of the owning app-config for this device |
|
|
Yes |
The UUID of the device that is affected by or has caused the event |
|
|
No |
The UUID of the session that this event belongs to (if any) |
|
|
No |
Status of the current event (SUCCESS or FAILURE) |
|
|
No |
Refers to details about the resource event (if available) |
Example request body
{
"event_id" : "f126589e-966f-4b22-a1a2-2b0b227c7b9b",
"subscribed_type" : "DEVICE_LOCKED",
"app_config_id" : "1b0dd485-c97f-4ca2-9dfd-f84fa5a24627",
"device_id" : "1b08c0fd-52eb-48e1-81dd-ff56a45be971",
"session_id" : "3b2a26f1-e8ee-4a5f-bdac-3da1ab40cd1e",
"ref" : "/devices/1b08c0fd-52eb-48e1-81dd-ff56a45be971/lock",
"status" : "SUCCESS"
}
Expected response from service provider
Return an empty response, with successful http code if the operation succeeds. Return a non 2xx http code object if something goes wrong.
Example request
$ curl 'http://localhost:8080/api/dummyspeventcallbackcontroller' -i -X POST \
-H 'Content-Type: application/json' \
-d '{
"event_id" : "f126589e-966f-4b22-a1a2-2b0b227c7b9b",
"subscribed_type" : "DEVICE_LOCKED",
"app_config_id" : "1b0dd485-c97f-4ca2-9dfd-f84fa5a24627",
"device_id" : "1b08c0fd-52eb-48e1-81dd-ff56a45be971",
"session_id" : "3b2a26f1-e8ee-4a5f-bdac-3da1ab40cd1e",
"ref" : "/devices/1b08c0fd-52eb-48e1-81dd-ff56a45be971/lock",
"status" : "SUCCESS"
}'
Example response
HTTP/1.1 204 No Content
6.2.3. Event callback status API
This provides a status overview of the event callback queues, including an availability-check of the event callback endpoint provided by the service provider. This will list the queue size for each app-config and perform an HTTP HEAD request towards the event callback endpoints configured.
Response objects types
Overall status object
Field | Type | Description |
---|---|---|
|
|
Overall queue health status |
|
|
List of event callback queues |
Queue details status object
Field | Type | Description |
---|---|---|
|
|
App-config id for the event callback queue |
|
|
Number of items in events callback queue |
|
|
Health status of the queue |
|
|
If 'status' is FAILURE, the error field should contain details about the failure |
Event-callback queue status
Status | Description |
---|---|
|
Queue health status is OK |
|
Queue status is unhealthy. An unreachable callback endpoint or a full callback queue will cause this error. |
Get API status
GET /api/admin/v1/status/callback
Example request
$ curl 'http://localhost:8080/api/admin/v1/status/callback' -i -u '85d8884c-c1f2-4bd1-b46c-0f0af07ae77a:3YBA6sfr+ukblV/aipnXpg==' -X GET
Example response - Status OK
HTTP/1.1 200 OK
X-Correlation-UUID: c64074ff-f2d4-4754-9f35-a8c4e5615a75
Content-Type: application/json
Content-Length: 137
{
"queues" : [ {
"id" : "cee947b1-eee3-42e5-9618-fab80be8928a",
"size" : 10,
"status" : "OK"
} ],
"status_all" : "OK"
}
Example response - Status FAILURE
HTTP/1.1 200 OK
X-Correlation-UUID: d8428ebb-2f03-467d-88c6-047fa3d762b3
Content-Type: application/json
Content-Length: 259
{
"queues" : [ {
"id" : "18755a96-2775-4287-b070-3819b4b592f4",
"size" : 10000,
"status" : "FAILURE",
"error" : "Queue size is larger than limit (5000) for app-config: 18755a96-2775-4287-b070-3819b4b592f4"
} ],
"status_all" : "FAILURE"
}